3 - Fixed handling of buffer boundaries in `format_to_n`
4 (https://github.com/fmtlib/fmt/issues/1996,
5 https://github.com/fmtlib/fmt/issues/2029).
6 - Fixed linkage errors when linking with a shared library
7 (https://github.com/fmtlib/fmt/issues/2011).
8 - Reintroduced ostream support to range formatters
9 (https://github.com/fmtlib/fmt/issues/2014).
10 - Worked around an issue with mixing std versions in gcc
11 (https://github.com/fmtlib/fmt/issues/2017).
15 - Fixed floating point formatting with large precision
16 (https://github.com/fmtlib/fmt/issues/1976).
20 - Fixed ABI compatibility with 7.0.x
21 (https://github.com/fmtlib/fmt/issues/1961).
22 - Added the `FMT_ARM_ABI_COMPATIBILITY` macro to work around ABI
23 incompatibility between GCC and Clang on ARM
24 (https://github.com/fmtlib/fmt/issues/1919).
25 - Worked around a SFINAE bug in GCC 8
26 (https://github.com/fmtlib/fmt/issues/1957).
27 - Fixed linkage errors when building with GCC\'s LTO
28 (https://github.com/fmtlib/fmt/issues/1955).
29 - Fixed a compilation error when building without `__builtin_clz` or
30 equivalent (https://github.com/fmtlib/fmt/pull/1968).
32 - Fixed a sign conversion warning
33 (https://github.com/fmtlib/fmt/pull/1964). Thanks @OptoCloud.
38 [Grisu3](https://www.cs.tufts.edu/~nr/cs257/archive/florian-loitsch/printf.pdf)
39 to [Dragonbox](https://github.com/jk-jeon/dragonbox) for the default
40 floating-point formatting which gives the shortest decimal
41 representation with round-trip guarantee and correct rounding
42 (https://github.com/fmtlib/fmt/pull/1882,
43 https://github.com/fmtlib/fmt/pull/1887,
44 https://github.com/fmtlib/fmt/pull/1894). This makes {fmt}
45 up to 20-30x faster than common implementations of
46 `std::ostringstream` and `sprintf` on
47 [dtoa-benchmark](https://github.com/fmtlib/dtoa-benchmark) and
48 faster than double-conversion and Ryƫ:
50 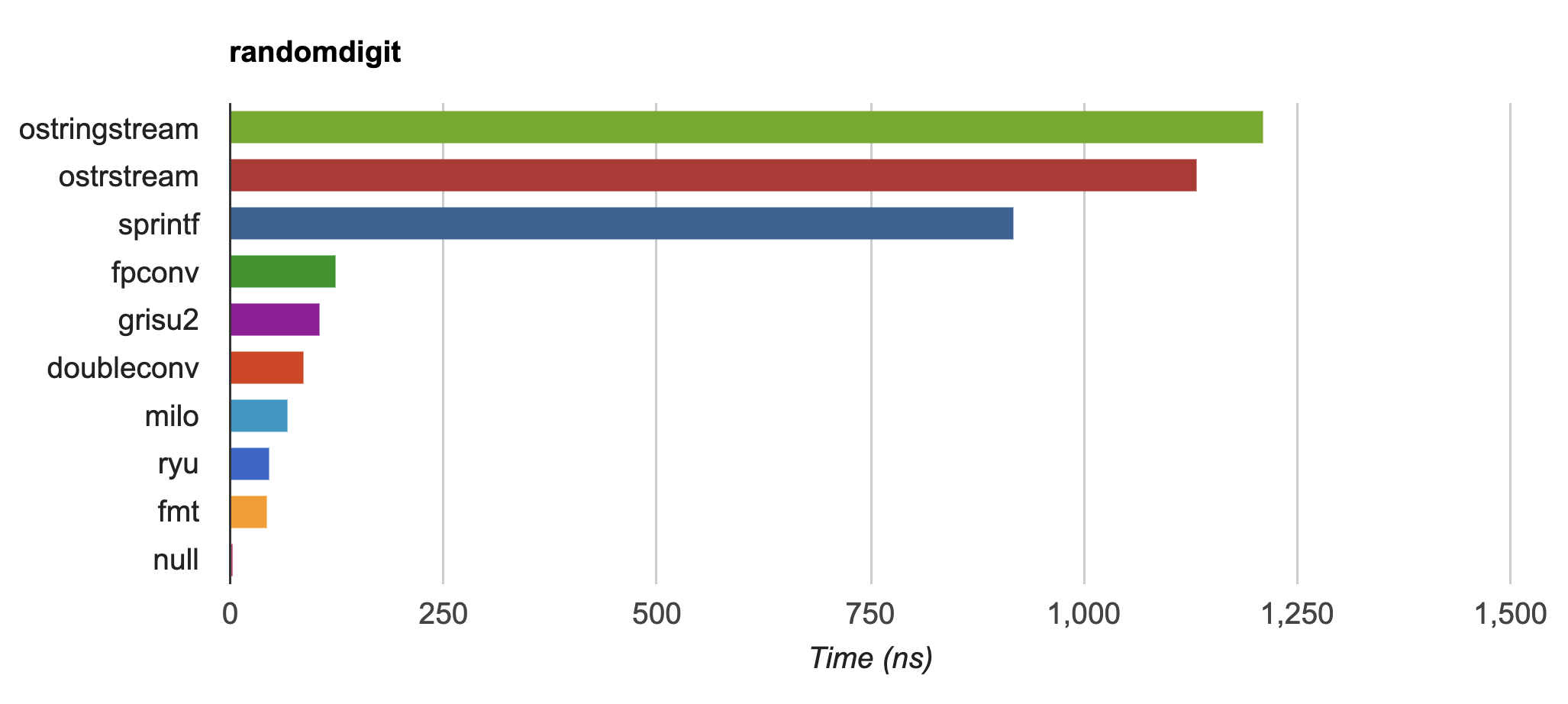
52 It is possible to get even better performance at the cost of larger
53 binary size by compiling with the `FMT_USE_FULL_CACHE_DRAGONBOX`
58 - Added an experimental unsynchronized file output API which, together
60 compilation](https://fmt.dev/latest/api.html#compile-api), can give
61 [5-9 times speed up compared to
62 fprintf](https://www.zverovich.net/2020/08/04/optimal-file-buffer-size.html)
63 on common platforms ([godbolt](https://godbolt.org/z/nsTcG8)):
69 auto f = fmt::output_file("guide");
70 f.print("The answer is {}.", 42);
74 - Added a formatter for `std::chrono::time_point<system_clock>`
75 (https://github.com/fmtlib/fmt/issues/1819,
76 https://github.com/fmtlib/fmt/pull/1837). For example
77 ([godbolt](https://godbolt.org/z/c4M6fh)):
80 #include <fmt/chrono.h>
83 auto now = std::chrono::system_clock::now();
84 fmt::print("The time is {:%H:%M:%S}.\n", now);
90 - Added support for ranges with non-const `begin`/`end` to `fmt::join`
91 (https://github.com/fmtlib/fmt/issues/1784,
92 https://github.com/fmtlib/fmt/pull/1786). For example
93 ([godbolt](https://godbolt.org/z/jP63Tv)):
96 #include <fmt/ranges.h>
97 #include <range/v3/view/filter.hpp>
100 using std::literals::string_literals::operator""s;
101 auto strs = std::array{"a"s, "bb"s, "ccc"s};
102 auto range = strs | ranges::views::filter(
103 [] (const std::string &x) { return x.size() != 2; }
105 fmt::print("{}\n", fmt::join(range, ""));
113 - Added a `memory_buffer::append` overload that takes a range
114 (https://github.com/fmtlib/fmt/pull/1806). Thanks @BRevzin.
116 - Improved handling of single code units in `FMT_COMPILE`. For
120 #include <fmt/compile.h>
123 return fmt::format_to(buf, FMT_COMPILE("x{}"), 42);
127 compiles to just ([godbolt](https://godbolt.org/z/5vncz3)):
133 cmpl $42, _ZN3fmt2v76detail10basic_dataIvE23zero_or_powers_of_10_32E+8(%rip)
137 movzwl _ZN3fmt2v76detail10basic_dataIvE6digitsE+84(%rip), %edx
144 Here a single `mov` instruction writes `'x'` (`$120`) to the output
147 - Added dynamic width support to format string compilation
148 (https://github.com/fmtlib/fmt/issues/1809).
150 - Improved error reporting for unformattable types: now you\'ll get
151 the type name directly in the error message instead of the note:
154 #include <fmt/core.h>
156 struct how_about_no {};
159 fmt::print("{}", how_about_no());
163 Error ([godbolt](https://godbolt.org/z/GoxM4e)):
165 `fmt/core.h:1438:3: error: static_assert failed due to requirement 'fmt::v7::formattable<how_about_no>()' "Cannot format an argument. To make type T formattable provide a formatter<T> specialization: https://fmt.dev/latest/api.html#udt" ...`
168 [make_args_checked](https://fmt.dev/7.1.0/api.html#argument-lists)
169 function template that allows you to write formatting functions with
170 compile-time format string checks and avoid binary code bloat
171 ([godbolt](https://godbolt.org/z/PEf9qr)):
174 void vlog(const char* file, int line, fmt::string_view format,
175 fmt::format_args args) {
176 fmt::print("{}: {}: ", file, line);
177 fmt::vprint(format, args);
180 template <typename S, typename... Args>
181 void log(const char* file, int line, const S& format, Args&&... args) {
182 vlog(file, line, format,
183 fmt::make_args_checked<Args...>(format, args...));
186 #define MY_LOG(format, ...) \
187 log(__FILE__, __LINE__, FMT_STRING(format), __VA_ARGS__)
189 MY_LOG("invalid squishiness: {}", 42);
192 - Replaced `snprintf` fallback with a faster internal IEEE 754 `float`
193 and `double` formatter for arbitrary precision. For example
194 ([godbolt](https://godbolt.org/z/dPhWvj)):
197 #include <fmt/core.h>
200 fmt::print("{:.500}\n", 4.9406564584124654E-324);
206 `4.9406564584124654417656879286822137236505980261432476442558568250067550727020875186529983636163599237979656469544571773092665671035593979639877479601078187812630071319031140452784581716784898210368871863605699873072305000638740915356498438731247339727316961514003171538539807412623856559117102665855668676818703956031062493194527159149245532930545654440112748012970999954193198940908041656332452475714786901472678015935523861155013480352649347201937902681071074917033322268447533357208324319360923829e-324`.
208 - Made `format_to_n` and `formatted_size` part of the [core
209 API](https://fmt.dev/latest/api.html#core-api)
210 ([godbolt](https://godbolt.org/z/sPjY1K)):
213 #include <fmt/core.h>
217 auto result = fmt::format_to_n(buffer, sizeof(buffer), "{}", 42);
221 - Added `fmt::format_to_n` overload with format string compilation
222 (https://github.com/fmtlib/fmt/issues/1764,
223 https://github.com/fmtlib/fmt/pull/1767,
224 https://github.com/fmtlib/fmt/pull/1869). For example
225 ([godbolt](https://godbolt.org/z/93h86q)):
228 #include <fmt/compile.h>
232 fmt::format_to_n(buffer, sizeof(buffer), FMT_COMPILE("{}"), 42);
236 Thanks @Kurkin and @alexezeder.
238 - Added `fmt::format_to` overload that take `text_style`
239 (https://github.com/fmtlib/fmt/issues/1593,
240 https://github.com/fmtlib/fmt/issues/1842,
241 https://github.com/fmtlib/fmt/pull/1843). For example
242 ([godbolt](https://godbolt.org/z/91153r)):
245 #include <fmt/color.h>
249 fmt::format_to(std::back_inserter(out),
250 fmt::emphasis::bold | fg(fmt::color::red),
251 "The answer is {}.", 42);
257 - Made the `'#'` specifier emit trailing zeros in addition to the
258 decimal point (https://github.com/fmtlib/fmt/issues/1797).
259 For example ([godbolt](https://godbolt.org/z/bhdcW9)):
262 #include <fmt/core.h>
265 fmt::print("{:#.2g}", 0.5);
271 - Changed the default floating point format to not include `.0` for
272 consistency with `std::format` and `std::to_chars`
273 (https://github.com/fmtlib/fmt/issues/1893,
274 https://github.com/fmtlib/fmt/issues/1943). It is possible
275 to get the decimal point and trailing zero with the `#` specifier.
277 - Fixed an issue with floating-point formatting that could result in
278 addition of a non-significant trailing zero in rare cases e.g.
279 `1.00e-34` instead of `1.0e-34`
280 (https://github.com/fmtlib/fmt/issues/1873,
281 https://github.com/fmtlib/fmt/issues/1917).
283 - Made `fmt::to_string` fallback on `ostream` insertion operator if
284 the `formatter` specialization is not provided
285 (https://github.com/fmtlib/fmt/issues/1815,
286 https://github.com/fmtlib/fmt/pull/1829). Thanks @alexezeder.
288 - Added support for the append mode to the experimental file API and
289 improved `fcntl.h` detection.
290 (https://github.com/fmtlib/fmt/pull/1847,
291 https://github.com/fmtlib/fmt/pull/1848). Thanks @t-wiser.
293 - Fixed handling of types that have both an implicit conversion
294 operator and an overloaded `ostream` insertion operator
295 (https://github.com/fmtlib/fmt/issues/1766).
297 - Fixed a slicing issue in an internal iterator type
298 (https://github.com/fmtlib/fmt/pull/1822). Thanks @BRevzin.
300 - Fixed an issue in locale-specific integer formatting
301 (https://github.com/fmtlib/fmt/issues/1927).
303 - Fixed handling of exotic code unit types
304 (https://github.com/fmtlib/fmt/issues/1870,
305 https://github.com/fmtlib/fmt/issues/1932).
307 - Improved `FMT_ALWAYS_INLINE`
308 (https://github.com/fmtlib/fmt/pull/1878). Thanks @jk-jeon.
310 - Removed dependency on `windows.h`
311 (https://github.com/fmtlib/fmt/pull/1900). Thanks @bernd5.
313 - Optimized counting of decimal digits on MSVC
314 (https://github.com/fmtlib/fmt/pull/1890). Thanks @mwinterb.
316 - Improved documentation
317 (https://github.com/fmtlib/fmt/issues/1772,
318 https://github.com/fmtlib/fmt/pull/1775,
319 https://github.com/fmtlib/fmt/pull/1792,
320 https://github.com/fmtlib/fmt/pull/1838,
321 https://github.com/fmtlib/fmt/pull/1888,
322 https://github.com/fmtlib/fmt/pull/1918,
323 https://github.com/fmtlib/fmt/pull/1939).
324 Thanks @leolchat, @pepsiman, @Klaim, @ravijanjam, @francesco-st and @udnaan.
326 - Added the `FMT_REDUCE_INT_INSTANTIATIONS` CMake option that reduces
327 the binary code size at the cost of some integer formatting
328 performance. This can be useful for extremely memory-constrained
330 (https://github.com/fmtlib/fmt/issues/1778,
331 https://github.com/fmtlib/fmt/pull/1781). Thanks @kammce.
333 - Added the `FMT_USE_INLINE_NAMESPACES` macro to control usage of
335 (https://github.com/fmtlib/fmt/pull/1945). Thanks @darklukee.
337 - Improved build configuration
338 (https://github.com/fmtlib/fmt/pull/1760,
339 https://github.com/fmtlib/fmt/pull/1770,
340 https://github.com/fmtlib/fmt/issues/1779,
341 https://github.com/fmtlib/fmt/pull/1783,
342 https://github.com/fmtlib/fmt/pull/1823).
343 Thanks @dvetutnev, @xvitaly, @tambry, @medithe and @martinwuehrer.
345 - Fixed various warnings and compilation issues
346 (https://github.com/fmtlib/fmt/pull/1790,
347 https://github.com/fmtlib/fmt/pull/1802,
348 https://github.com/fmtlib/fmt/pull/1808,
349 https://github.com/fmtlib/fmt/issues/1810,
350 https://github.com/fmtlib/fmt/issues/1811,
351 https://github.com/fmtlib/fmt/pull/1812,
352 https://github.com/fmtlib/fmt/pull/1814,
353 https://github.com/fmtlib/fmt/pull/1816,
354 https://github.com/fmtlib/fmt/pull/1817,
355 https://github.com/fmtlib/fmt/pull/1818,
356 https://github.com/fmtlib/fmt/issues/1825,
357 https://github.com/fmtlib/fmt/pull/1836,
358 https://github.com/fmtlib/fmt/pull/1855,
359 https://github.com/fmtlib/fmt/pull/1856,
360 https://github.com/fmtlib/fmt/pull/1860,
361 https://github.com/fmtlib/fmt/pull/1877,
362 https://github.com/fmtlib/fmt/pull/1879,
363 https://github.com/fmtlib/fmt/pull/1880,
364 https://github.com/fmtlib/fmt/issues/1896,
365 https://github.com/fmtlib/fmt/pull/1897,
366 https://github.com/fmtlib/fmt/pull/1898,
367 https://github.com/fmtlib/fmt/issues/1904,
368 https://github.com/fmtlib/fmt/pull/1908,
369 https://github.com/fmtlib/fmt/issues/1911,
370 https://github.com/fmtlib/fmt/issues/1912,
371 https://github.com/fmtlib/fmt/issues/1928,
372 https://github.com/fmtlib/fmt/pull/1929,
373 https://github.com/fmtlib/fmt/issues/1935,
374 https://github.com/fmtlib/fmt/pull/1937,
375 https://github.com/fmtlib/fmt/pull/1942,
376 https://github.com/fmtlib/fmt/issues/1949).
377 Thanks @TheQwertiest, @medithe, @martinwuehrer, @n16h7hunt3r, @Othereum,
378 @gsjaardema, @AlexanderLanin, @gcerretani, @chronoxor, @noizefloor,
379 @akohlmey, @jk-jeon, @rimathia, @rglarix, @moiwi, @heckad, @MarcDirven.
380 @BartSiwek and @darklukee.
384 - Worked around broken `numeric_limits` for 128-bit integers
385 (https://github.com/fmtlib/fmt/issues/1787).
386 - Added error reporting on missing named arguments
387 (https://github.com/fmtlib/fmt/issues/1796).
388 - Stopped using 128-bit integers with clang-cl
389 (https://github.com/fmtlib/fmt/pull/1800). Thanks @Kingcom.
390 - Fixed issues in locale-specific integer formatting
391 (https://github.com/fmtlib/fmt/issues/1782,
392 https://github.com/fmtlib/fmt/issues/1801).
396 - Worked around broken `numeric_limits` for 128-bit integers
397 (https://github.com/fmtlib/fmt/issues/1725).
398 - Fixed compatibility with CMake 3.4
399 (https://github.com/fmtlib/fmt/issues/1779).
400 - Fixed handling of digit separators in locale-specific formatting
401 (https://github.com/fmtlib/fmt/issues/1782).
405 - Updated the inline version namespace name.
406 - Worked around a gcc bug in mangling of alias templates
407 (https://github.com/fmtlib/fmt/issues/1753).
408 - Fixed a linkage error on Windows
409 (https://github.com/fmtlib/fmt/issues/1757). Thanks @Kurkin.
410 - Fixed minor issues with the documentation.
414 - Reduced the library size. For example, on macOS a stripped test
415 binary statically linked with {fmt} [shrank from \~368k to less than
416 100k](http://www.zverovich.net/2020/05/21/reducing-library-size.html).
418 - Added a simpler and more efficient [format string compilation
419 API](https://fmt.dev/7.0.0/api.html#compile-api):
422 #include <fmt/compile.h>
424 // Converts 42 into std::string using the most efficient method and no
425 // runtime format string processing.
426 std::string s = fmt::format(FMT_COMPILE("{}"), 42);
429 The old `fmt::compile` API is now deprecated.
431 - Optimized integer formatting: `format_to` with format string
432 compilation and a stack-allocated buffer is now [faster than
433 to_chars on both libc++ and
434 libstdc++](http://www.zverovich.net/2020/06/13/fast-int-to-string-revisited.html).
436 - Optimized handling of small format strings. For example,
439 fmt::format("Result: {}: ({},{},{},{})", str1, str2, str3, str4, str5)
443 (https://github.com/fmtlib/fmt/issues/1685).
445 - Applied extern templates to improve compile times when using the
446 core API and `fmt/format.h`
447 (https://github.com/fmtlib/fmt/issues/1452). For example,
448 on macOS with clang the compile time of a test translation unit
449 dropped from 2.3s to 0.3s with `-O2` and from 0.6s to 0.3s with the
450 default settings (`-O0`).
454 % time c++ -c test.cc -I include -std=c++17 -O2
455 c++ -c test.cc -I include -std=c++17 -O2 2.22s user 0.08s system 99% cpu 2.311 total
459 % time c++ -c test.cc -I include -std=c++17 -O2
460 c++ -c test.cc -I include -std=c++17 -O2 0.26s user 0.04s system 98% cpu 0.303 total
464 % time c++ -c test.cc -I include -std=c++17
465 c++ -c test.cc -I include -std=c++17 0.53s user 0.06s system 98% cpu 0.601 total
469 % time c++ -c test.cc -I include -std=c++17
470 c++ -c test.cc -I include -std=c++17 0.24s user 0.06s system 98% cpu 0.301 total
472 It is still recommended to use `fmt/core.h` instead of
473 `fmt/format.h` but the compile time difference is now smaller.
474 Thanks @alex3d for the suggestion.
476 - Named arguments are now stored on stack (no dynamic memory
477 allocations) and the compiled code is more compact and efficient.
481 #include <fmt/core.h>
484 fmt::print("The answer is {answer}\n", fmt::arg("answer", 42));
488 compiles to just ([godbolt](https://godbolt.org/z/NcfEp_))
494 .string "The answer is {answer}\n"
497 mov edi, OFFSET FLAT:.LC1
499 movabs rdx, 4611686018427387905
502 mov QWORD PTR [rsp+8], 1
503 mov QWORD PTR [rsp], rax
504 mov DWORD PTR [rsp+16], 42
505 mov QWORD PTR [rsp+32], OFFSET FLAT:.LC0
506 mov DWORD PTR [rsp+40], 0
507 call fmt::v6::vprint(fmt::v6::basic_string_view<char>,
508 fmt::v6::format_args)
517 - Implemented compile-time checks for dynamic width and precision
518 (https://github.com/fmtlib/fmt/issues/1614):
521 #include <fmt/format.h>
524 fmt::print(FMT_STRING("{0:{1}}"), 42);
528 now gives a compilation error because argument 1 doesn\'t exist:
530 In file included from test.cc:1:
531 include/fmt/format.h:2726:27: error: constexpr variable 'invalid_format' must be
532 initialized by a constant expression
533 FMT_CONSTEXPR_DECL bool invalid_format =
536 include/fmt/core.h:569:26: note: in call to
537 '&checker(s, {}).context_->on_error(&"argument not found"[0])'
538 if (id >= num_args_) on_error("argument not found");
541 - Added sentinel support to `fmt::join`
542 (https://github.com/fmtlib/fmt/pull/1689)
545 struct zstring_sentinel {};
546 bool operator==(const char* p, zstring_sentinel) { return *p == '\0'; }
547 bool operator!=(const char* p, zstring_sentinel) { return *p != '\0'; }
551 const char* begin() const { return p; }
552 zstring_sentinel end() const { return {}; }
555 auto s = fmt::format("{}", fmt::join(zstring{"hello"}, "_"));
561 - Added support for named arguments, `clear` and `reserve` to
562 `dynamic_format_arg_store`
563 (https://github.com/fmtlib/fmt/issues/1655,
564 https://github.com/fmtlib/fmt/pull/1663,
565 https://github.com/fmtlib/fmt/pull/1674,
566 https://github.com/fmtlib/fmt/pull/1677). Thanks @vsolontsov-ll.
568 - Added support for the `'c'` format specifier to integral types for
569 compatibility with `std::format`
570 (https://github.com/fmtlib/fmt/issues/1652).
572 - Replaced the `'n'` format specifier with `'L'` for compatibility
574 (https://github.com/fmtlib/fmt/issues/1624). The `'n'`
575 specifier can be enabled via the `FMT_DEPRECATED_N_SPECIFIER` macro.
577 - The `'='` format specifier is now disabled by default for
578 compatibility with `std::format`. It can be enabled via the
579 `FMT_DEPRECATED_NUMERIC_ALIGN` macro.
581 - Removed the following deprecated APIs:
583 - `FMT_STRING_ALIAS` and `fmt` macros - replaced by `FMT_STRING`
584 - `fmt::basic_string_view::char_type` - replaced by
585 `fmt::basic_string_view::value_type`
587 - `format_arg_store::types`
588 - `*parse_context` - replaced by `*format_parse_context`
589 - `FMT_DEPRECATED_INCLUDE_OS`
590 - `FMT_DEPRECATED_PERCENT` - incompatible with `std::format`
591 - `*writer` - replaced by compiled format API
593 - Renamed the `internal` namespace to `detail`
594 (https://github.com/fmtlib/fmt/issues/1538). The former is
595 still provided as an alias if the `FMT_USE_INTERNAL` macro is
598 - Improved compatibility between `fmt::printf` with the standard specs
599 (https://github.com/fmtlib/fmt/issues/1595,
600 https://github.com/fmtlib/fmt/pull/1682,
601 https://github.com/fmtlib/fmt/pull/1683,
602 https://github.com/fmtlib/fmt/pull/1687,
603 https://github.com/fmtlib/fmt/pull/1699). Thanks @rimathia.
605 - Fixed handling of `operator<<` overloads that use `copyfmt`
606 (https://github.com/fmtlib/fmt/issues/1666).
608 - Added the `FMT_OS` CMake option to control inclusion of OS-specific
609 APIs in the fmt target. This can be useful for embedded platforms
610 (https://github.com/fmtlib/fmt/issues/1654,
611 https://github.com/fmtlib/fmt/pull/1656). Thanks @kwesolowski.
613 - Replaced `FUZZING_BUILD_MODE_UNSAFE_FOR_PRODUCTION` with the
614 `FMT_FUZZ` macro to prevent interfering with fuzzing of projects
615 using {fmt} (https://github.com/fmtlib/fmt/pull/1650).
618 - Fixed compatibility with emscripten
619 (https://github.com/fmtlib/fmt/issues/1636,
620 https://github.com/fmtlib/fmt/pull/1637). Thanks @ArthurSonzogni.
622 - Improved documentation
623 (https://github.com/fmtlib/fmt/issues/704,
624 https://github.com/fmtlib/fmt/pull/1643,
625 https://github.com/fmtlib/fmt/pull/1660,
626 https://github.com/fmtlib/fmt/pull/1681,
627 https://github.com/fmtlib/fmt/pull/1691,
628 https://github.com/fmtlib/fmt/pull/1706,
629 https://github.com/fmtlib/fmt/pull/1714,
630 https://github.com/fmtlib/fmt/pull/1721,
631 https://github.com/fmtlib/fmt/pull/1739,
632 https://github.com/fmtlib/fmt/pull/1740,
633 https://github.com/fmtlib/fmt/pull/1741,
634 https://github.com/fmtlib/fmt/pull/1751).
635 Thanks @senior7515, @lsr0, @puetzk, @fpelliccioni, Alexey Kuzmenko, @jelly,
636 @claremacrae, @jiapengwen, @gsjaardema and @alexey-milovidov.
638 - Implemented various build configuration fixes and improvements
639 (https://github.com/fmtlib/fmt/pull/1603,
640 https://github.com/fmtlib/fmt/pull/1657,
641 https://github.com/fmtlib/fmt/pull/1702,
642 https://github.com/fmtlib/fmt/pull/1728).
643 Thanks @scramsby, @jtojnar, @orivej and @flagarde.
645 - Fixed various warnings and compilation issues
646 (https://github.com/fmtlib/fmt/pull/1616,
647 https://github.com/fmtlib/fmt/issues/1620,
648 https://github.com/fmtlib/fmt/issues/1622,
649 https://github.com/fmtlib/fmt/issues/1625,
650 https://github.com/fmtlib/fmt/pull/1627,
651 https://github.com/fmtlib/fmt/issues/1628,
652 https://github.com/fmtlib/fmt/pull/1629,
653 https://github.com/fmtlib/fmt/issues/1631,
654 https://github.com/fmtlib/fmt/pull/1633,
655 https://github.com/fmtlib/fmt/pull/1649,
656 https://github.com/fmtlib/fmt/issues/1658,
657 https://github.com/fmtlib/fmt/pull/1661,
658 https://github.com/fmtlib/fmt/pull/1667,
659 https://github.com/fmtlib/fmt/issues/1668,
660 https://github.com/fmtlib/fmt/pull/1669,
661 https://github.com/fmtlib/fmt/issues/1692,
662 https://github.com/fmtlib/fmt/pull/1696,
663 https://github.com/fmtlib/fmt/pull/1697,
664 https://github.com/fmtlib/fmt/issues/1707,
665 https://github.com/fmtlib/fmt/pull/1712,
666 https://github.com/fmtlib/fmt/pull/1716,
667 https://github.com/fmtlib/fmt/pull/1722,
668 https://github.com/fmtlib/fmt/issues/1724,
669 https://github.com/fmtlib/fmt/pull/1729,
670 https://github.com/fmtlib/fmt/pull/1738,
671 https://github.com/fmtlib/fmt/issues/1742,
672 https://github.com/fmtlib/fmt/issues/1743,
673 https://github.com/fmtlib/fmt/pull/1744,
674 https://github.com/fmtlib/fmt/issues/1747,
675 https://github.com/fmtlib/fmt/pull/1750).
676 Thanks @gsjaardema, @gabime, @johnor, @Kurkin, @invexed, @peterbell10,
677 @daixtrose, @petrutlucian94, @Neargye, @ambitslix, @gabime, @erthink,
678 @tohammer and @0x8000-0000.
682 - Fixed ostream support in `sprintf`
683 (https://github.com/fmtlib/fmt/issues/1631).
684 - Fixed type detection when using implicit conversion to `string_view`
685 and ostream `operator<<` inconsistently
686 (https://github.com/fmtlib/fmt/issues/1662).
690 - Improved error reporting when trying to format an object of a
691 non-formattable type:
694 fmt::format("{}", S());
699 include/fmt/core.h:1015:5: error: static_assert failed due to requirement
700 'formattable' "Cannot format argument. To make type T formattable provide a
701 formatter<T> specialization:
702 https://fmt.dev/latest/api.html#formatting-user-defined-types"
706 note: in instantiation of function template specialization
707 'fmt::v6::format<char [3], S, char>' requested here
708 fmt::format("{}", S());
711 if `S` is not formattable.
713 - Reduced the library size by \~10%.
715 - Always print decimal point if `#` is specified
716 (https://github.com/fmtlib/fmt/issues/1476,
717 https://github.com/fmtlib/fmt/issues/1498):
720 fmt::print("{:#.0f}", 42.0);
725 - Implemented the `'L'` specifier for locale-specific numeric
726 formatting to improve compatibility with `std::format`. The `'n'`
727 specifier is now deprecated and will be removed in the next major
730 - Moved OS-specific APIs such as `windows_error` from `fmt/format.h`
731 to `fmt/os.h`. You can define `FMT_DEPRECATED_INCLUDE_OS` to
732 automatically include `fmt/os.h` from `fmt/format.h` for
733 compatibility but this will be disabled in the next major release.
735 - Added precision overflow detection in floating-point formatting.
737 - Implemented detection of invalid use of `fmt::arg`.
739 - Used `type_identity` to block unnecessary template argument
740 deduction. Thanks Tim Song.
742 - Improved UTF-8 handling
743 (https://github.com/fmtlib/fmt/issues/1109):
746 fmt::print("â{0:â^{2}}â\n"
748 "â{0:â^{2}}â\n", "", "ĐŃŃĐČŃŃĐ°ĐœĐœĐ”, ŃĐČĐ”Ń!", 21);
753 âââââââââââââââââââââââ
754 â ĐŃŃĐČŃŃĐ°ĐœĐœĐ”, ŃĐČĐ”Ń! â
755 âââââââââââââââââââââââ
757 on systems that support Unicode.
759 - Added experimental dynamic argument storage
760 (https://github.com/fmtlib/fmt/issues/1170,
761 https://github.com/fmtlib/fmt/pull/1584):
764 fmt::dynamic_format_arg_store<fmt::format_context> store;
765 store.push_back("answer");
767 fmt::vprint("The {} is {}.\n", store);
774 Thanks @vsolontsov-ll.
776 - Made `fmt::join` accept `initializer_list`
777 (https://github.com/fmtlib/fmt/pull/1591). Thanks @Rapotkinnik.
779 - Fixed handling of empty tuples
780 (https://github.com/fmtlib/fmt/issues/1588).
782 - Fixed handling of output iterators in `format_to_n`
783 (https://github.com/fmtlib/fmt/issues/1506).
785 - Fixed formatting of `std::chrono::duration` types to wide output
786 (https://github.com/fmtlib/fmt/pull/1533). Thanks @zeffy.
788 - Added const `begin` and `end` overload to buffers
789 (https://github.com/fmtlib/fmt/pull/1553). Thanks @dominicpoeschko.
791 - Added the ability to disable floating-point formatting via
792 `FMT_USE_FLOAT`, `FMT_USE_DOUBLE` and `FMT_USE_LONG_DOUBLE` macros
793 for extremely memory-constrained embedded system
794 (https://github.com/fmtlib/fmt/pull/1590). Thanks @albaguirre.
796 - Made `FMT_STRING` work with `constexpr` `string_view`
797 (https://github.com/fmtlib/fmt/pull/1589). Thanks @scramsby.
799 - Implemented a minor optimization in the format string parser
800 (https://github.com/fmtlib/fmt/pull/1560). Thanks @IkarusDeveloper.
802 - Improved attribute detection
803 (https://github.com/fmtlib/fmt/pull/1469,
804 https://github.com/fmtlib/fmt/pull/1475,
805 https://github.com/fmtlib/fmt/pull/1576).
806 Thanks @federico-busato, @chronoxor and @refnum.
808 - Improved documentation
809 (https://github.com/fmtlib/fmt/pull/1481,
810 https://github.com/fmtlib/fmt/pull/1523).
811 Thanks @JackBoosY and @imba-tjd.
813 - Fixed symbol visibility on Linux when compiling with
814 `-fvisibility=hidden`
815 (https://github.com/fmtlib/fmt/pull/1535). Thanks @milianw.
817 - Implemented various build configuration fixes and improvements
818 (https://github.com/fmtlib/fmt/issues/1264,
819 https://github.com/fmtlib/fmt/issues/1460,
820 https://github.com/fmtlib/fmt/pull/1534,
821 https://github.com/fmtlib/fmt/issues/1536,
822 https://github.com/fmtlib/fmt/issues/1545,
823 https://github.com/fmtlib/fmt/pull/1546,
824 https://github.com/fmtlib/fmt/issues/1566,
825 https://github.com/fmtlib/fmt/pull/1582,
826 https://github.com/fmtlib/fmt/issues/1597,
827 https://github.com/fmtlib/fmt/pull/1598).
828 Thanks @ambitslix, @jwillikers and @stac47.
830 - Fixed various warnings and compilation issues
831 (https://github.com/fmtlib/fmt/pull/1433,
832 https://github.com/fmtlib/fmt/issues/1461,
833 https://github.com/fmtlib/fmt/pull/1470,
834 https://github.com/fmtlib/fmt/pull/1480,
835 https://github.com/fmtlib/fmt/pull/1485,
836 https://github.com/fmtlib/fmt/pull/1492,
837 https://github.com/fmtlib/fmt/issues/1493,
838 https://github.com/fmtlib/fmt/issues/1504,
839 https://github.com/fmtlib/fmt/pull/1505,
840 https://github.com/fmtlib/fmt/pull/1512,
841 https://github.com/fmtlib/fmt/issues/1515,
842 https://github.com/fmtlib/fmt/pull/1516,
843 https://github.com/fmtlib/fmt/pull/1518,
844 https://github.com/fmtlib/fmt/pull/1519,
845 https://github.com/fmtlib/fmt/pull/1520,
846 https://github.com/fmtlib/fmt/pull/1521,
847 https://github.com/fmtlib/fmt/pull/1522,
848 https://github.com/fmtlib/fmt/issues/1524,
849 https://github.com/fmtlib/fmt/pull/1530,
850 https://github.com/fmtlib/fmt/issues/1531,
851 https://github.com/fmtlib/fmt/pull/1532,
852 https://github.com/fmtlib/fmt/issues/1539,
853 https://github.com/fmtlib/fmt/issues/1547,
854 https://github.com/fmtlib/fmt/issues/1548,
855 https://github.com/fmtlib/fmt/pull/1554,
856 https://github.com/fmtlib/fmt/issues/1567,
857 https://github.com/fmtlib/fmt/pull/1568,
858 https://github.com/fmtlib/fmt/pull/1569,
859 https://github.com/fmtlib/fmt/pull/1571,
860 https://github.com/fmtlib/fmt/pull/1573,
861 https://github.com/fmtlib/fmt/pull/1575,
862 https://github.com/fmtlib/fmt/pull/1581,
863 https://github.com/fmtlib/fmt/issues/1583,
864 https://github.com/fmtlib/fmt/issues/1586,
865 https://github.com/fmtlib/fmt/issues/1587,
866 https://github.com/fmtlib/fmt/issues/1594,
867 https://github.com/fmtlib/fmt/pull/1596,
868 https://github.com/fmtlib/fmt/issues/1604,
869 https://github.com/fmtlib/fmt/pull/1606,
870 https://github.com/fmtlib/fmt/issues/1607,
871 https://github.com/fmtlib/fmt/issues/1609).
872 Thanks @marti4d, @iPherian, @parkertomatoes, @gsjaardema, @chronoxor,
873 @DanielaE, @torsten48, @tohammer, @lefticus, @ryusakki, @adnsv, @fghzxm,
874 @refnum, @pramodk, @Spirrwell and @scramsby.
878 - Fixed ABI compatibility with `libfmt.so.6.0.0`
879 (https://github.com/fmtlib/fmt/issues/1471).
880 - Fixed handling types convertible to `std::string_view`
881 (https://github.com/fmtlib/fmt/pull/1451). Thanks @denizevrenci.
882 - Made CUDA test an opt-in enabled via the `FMT_CUDA_TEST` CMake
884 - Fixed sign conversion warnings
885 (https://github.com/fmtlib/fmt/pull/1440). Thanks @0x8000-0000.
889 - Fixed shared library build on Windows
890 (https://github.com/fmtlib/fmt/pull/1443,
891 https://github.com/fmtlib/fmt/issues/1445,
892 https://github.com/fmtlib/fmt/pull/1446,
893 https://github.com/fmtlib/fmt/issues/1450).
894 Thanks @egorpugin and @bbolli.
895 - Added a missing decimal point in exponent notation with trailing
897 - Removed deprecated `format_arg_store::TYPES`.
901 - {fmt} now formats IEEE 754 `float` and `double` using the shortest
902 decimal representation with correct rounding by default:
906 #include <fmt/core.h>
909 fmt::print("{}", M_PI);
913 prints `3.141592653589793`.
915 - Made the fast binary to decimal floating-point formatter the
916 default, simplified it and improved performance. {fmt} is now 15
917 times faster than libc++\'s `std::ostringstream`, 11 times faster
918 than `printf` and 10% faster than double-conversion on
919 [dtoa-benchmark](https://github.com/fmtlib/dtoa-benchmark):
921 | Function | Time (ns) | Speedup |
922 | ------------- | --------: | ------: |
923 | ostringstream | 1,346.30 | 1.00x |
924 | ostrstream | 1,195.74 | 1.13x |
925 | sprintf | 995.08 | 1.35x |
926 | doubleconv | 99.10 | 13.59x |
927 | fmt | 88.34 | 15.24x |
929 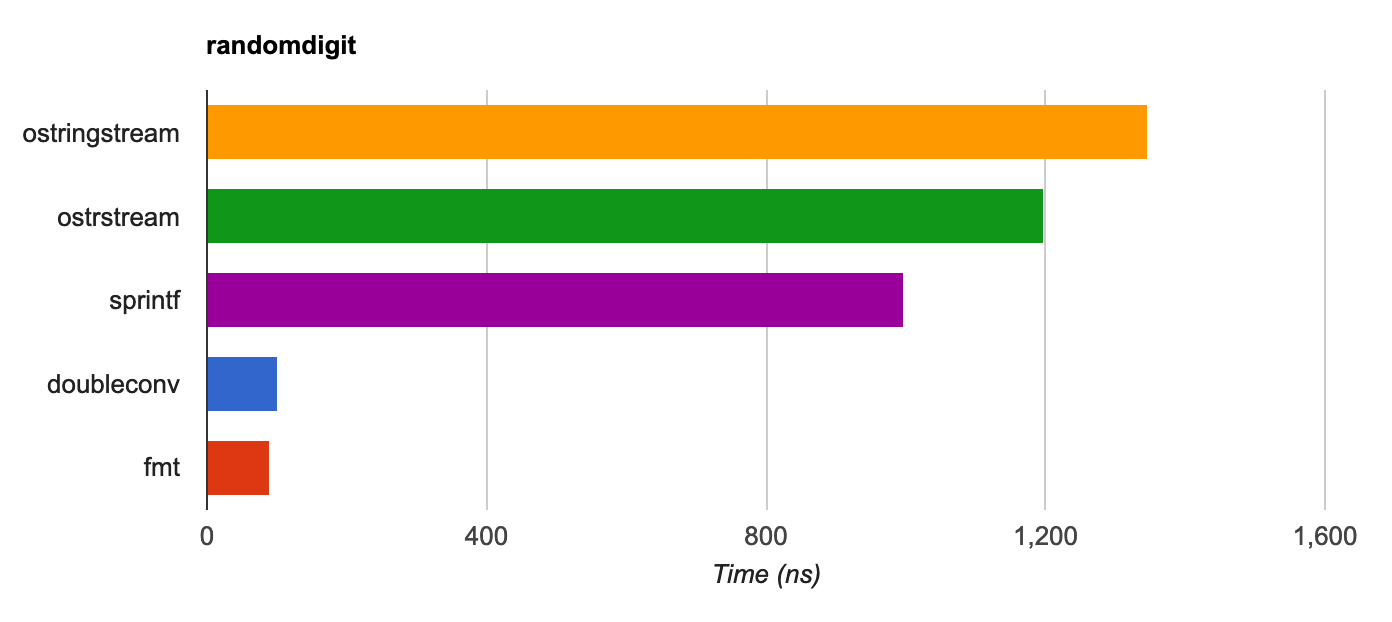
931 - {fmt} no longer converts `float` arguments to `double`. In
932 particular this improves the default (shortest) representation of
933 floats and makes `fmt::format` consistent with `std::format` specs
934 (https://github.com/fmtlib/fmt/issues/1336,
935 https://github.com/fmtlib/fmt/issues/1353,
936 https://github.com/fmtlib/fmt/pull/1360,
937 https://github.com/fmtlib/fmt/pull/1361):
940 fmt::print("{}", 0.1f);
943 prints `0.1` instead of `0.10000000149011612`.
947 - Made floating-point formatting output consistent with
949 (https://github.com/fmtlib/fmt/issues/1376,
950 https://github.com/fmtlib/fmt/issues/1417).
952 - Added support for 128-bit integers
953 (https://github.com/fmtlib/fmt/pull/1287):
956 fmt::print("{}", std::numeric_limits<__int128_t>::max());
959 prints `170141183460469231731687303715884105727`.
961 Thanks @denizevrenci.
963 - The overload of `print` that takes `text_style` is now atomic, i.e.
964 the output from different threads doesn\'t interleave
965 (https://github.com/fmtlib/fmt/pull/1351). Thanks @tankiJong.
967 - Made compile time in the header-only mode \~20% faster by reducing
968 the number of template instantiations. `wchar_t` overload of
969 `vprint` was moved from `fmt/core.h` to `fmt/format.h`.
971 - Added an overload of `fmt::join` that works with tuples
972 (https://github.com/fmtlib/fmt/issues/1322,
973 https://github.com/fmtlib/fmt/pull/1330):
977 #include <fmt/ranges.h>
980 std::tuple<char, int, float> t{'a', 1, 2.0f};
985 prints `('a', 1, 2.0)`.
989 - Changed formatting of octal zero with prefix from \"00\" to \"0\":
992 fmt::print("{:#o}", 0);
997 - The locale is now passed to ostream insertion (`<<`) operators
998 (https://github.com/fmtlib/fmt/pull/1406):
1001 #include <fmt/locale.h>
1002 #include <fmt/ostream.h>
1008 std::ostream& operator<<(std::ostream& os, S s) {
1009 return os << s.value;
1013 auto s = fmt::format(std::locale("fr_FR.UTF-8"), "{}", S{0.42});
1020 - Locale-specific number formatting now uses grouping
1021 (https://github.com/fmtlib/fmt/issues/1393,
1022 https://github.com/fmtlib/fmt/pull/1394). Thanks @skrdaniel.
1024 - Fixed handling of types with deleted implicit rvalue conversion to
1025 `const char**` (https://github.com/fmtlib/fmt/issues/1421):
1029 operator const char*() const&;
1030 operator const char*() &;
1031 operator const char*() const&& = delete;
1032 operator const char*() && = delete;
1035 fmt::print("{}", str); // now compiles
1038 - Enums are now mapped to correct underlying types instead of `int`
1039 (https://github.com/fmtlib/fmt/pull/1286). Thanks @agmt.
1041 - Enum classes are no longer implicitly converted to `int`
1042 (https://github.com/fmtlib/fmt/issues/1424).
1044 - Added `basic_format_parse_context` for consistency with C++20
1045 `std::format` and deprecated `basic_parse_context`.
1047 - Fixed handling of UTF-8 in precision
1048 (https://github.com/fmtlib/fmt/issues/1389,
1049 https://github.com/fmtlib/fmt/pull/1390). Thanks @tajtiattila.
1051 - {fmt} can now be installed on Linux, macOS and Windows with
1052 [Conda](https://docs.conda.io/en/latest/) using its
1053 [conda-forge](https://conda-forge.org)
1054 [package](https://github.com/conda-forge/fmt-feedstock)
1055 (https://github.com/fmtlib/fmt/pull/1410):
1057 conda install -c conda-forge fmt
1061 - Added a CUDA test (https://github.com/fmtlib/fmt/pull/1285,
1062 https://github.com/fmtlib/fmt/pull/1317).
1063 Thanks @luncliff and @risa2000.
1065 - Improved documentation
1066 (https://github.com/fmtlib/fmt/pull/1276,
1067 https://github.com/fmtlib/fmt/issues/1291,
1068 https://github.com/fmtlib/fmt/issues/1296,
1069 https://github.com/fmtlib/fmt/pull/1315,
1070 https://github.com/fmtlib/fmt/pull/1332,
1071 https://github.com/fmtlib/fmt/pull/1337,
1072 https://github.com/fmtlib/fmt/issues/1395
1073 https://github.com/fmtlib/fmt/pull/1418).
1074 Thanks @waywardmonkeys, @pauldreik and @jackoalan.
1076 - Various code improvements
1077 (https://github.com/fmtlib/fmt/pull/1358,
1078 https://github.com/fmtlib/fmt/pull/1407).
1079 Thanks @orivej and @dpacbach.
1081 - Fixed compile-time format string checks for user-defined types
1082 (https://github.com/fmtlib/fmt/issues/1292).
1084 - Worked around a false positive in `unsigned-integer-overflow` sanitizer
1085 (https://github.com/fmtlib/fmt/issues/1377).
1087 - Fixed various warnings and compilation issues
1088 (https://github.com/fmtlib/fmt/issues/1273,
1089 https://github.com/fmtlib/fmt/pull/1278,
1090 https://github.com/fmtlib/fmt/pull/1280,
1091 https://github.com/fmtlib/fmt/issues/1281,
1092 https://github.com/fmtlib/fmt/issues/1288,
1093 https://github.com/fmtlib/fmt/pull/1290,
1094 https://github.com/fmtlib/fmt/pull/1301,
1095 https://github.com/fmtlib/fmt/issues/1305,
1096 https://github.com/fmtlib/fmt/issues/1306,
1097 https://github.com/fmtlib/fmt/issues/1309,
1098 https://github.com/fmtlib/fmt/pull/1312,
1099 https://github.com/fmtlib/fmt/issues/1313,
1100 https://github.com/fmtlib/fmt/issues/1316,
1101 https://github.com/fmtlib/fmt/issues/1319,
1102 https://github.com/fmtlib/fmt/pull/1320,
1103 https://github.com/fmtlib/fmt/pull/1326,
1104 https://github.com/fmtlib/fmt/pull/1328,
1105 https://github.com/fmtlib/fmt/issues/1344,
1106 https://github.com/fmtlib/fmt/pull/1345,
1107 https://github.com/fmtlib/fmt/pull/1347,
1108 https://github.com/fmtlib/fmt/pull/1349,
1109 https://github.com/fmtlib/fmt/issues/1354,
1110 https://github.com/fmtlib/fmt/issues/1362,
1111 https://github.com/fmtlib/fmt/issues/1366,
1112 https://github.com/fmtlib/fmt/pull/1364,
1113 https://github.com/fmtlib/fmt/pull/1370,
1114 https://github.com/fmtlib/fmt/pull/1371,
1115 https://github.com/fmtlib/fmt/issues/1385,
1116 https://github.com/fmtlib/fmt/issues/1388,
1117 https://github.com/fmtlib/fmt/pull/1397,
1118 https://github.com/fmtlib/fmt/pull/1414,
1119 https://github.com/fmtlib/fmt/pull/1416,
1120 https://github.com/fmtlib/fmt/issues/1422
1121 https://github.com/fmtlib/fmt/pull/1427,
1122 https://github.com/fmtlib/fmt/issues/1431,
1123 https://github.com/fmtlib/fmt/pull/1433).
1124 Thanks @hhb, @gsjaardema, @gabime, @neheb, @vedranmiletic, @dkavolis,
1125 @mwinterb, @orivej, @denizevrenci, @leonklingele, @chronoxor, @kent-tri,
1126 @0x8000-0000 and @marti4d.
1128 # 6.0.0 - 2019-08-26
1130 - Switched to the [MIT license](
1131 https://github.com/fmtlib/fmt/blob/5a4b24613ba16cc689977c3b5bd8274a3ba1dd1f/LICENSE.rst)
1132 with an optional exception that allows distributing binary code
1133 without attribution.
1135 - Floating-point formatting is now locale-independent by default:
1139 #include <fmt/core.h>
1142 std::locale::global(std::locale("ru_RU.UTF-8"));
1143 fmt::print("value = {}", 4.2);
1147 prints \"value = 4.2\" regardless of the locale.
1149 For locale-specific formatting use the `n` specifier:
1152 std::locale::global(std::locale("ru_RU.UTF-8"));
1153 fmt::print("value = {:n}", 4.2);
1156 prints \"value = 4,2\".
1158 - Added an experimental Grisu floating-point formatting algorithm
1159 implementation (disabled by default). To enable it compile with the
1160 `FMT_USE_GRISU` macro defined to 1:
1163 #define FMT_USE_GRISU 1
1164 #include <fmt/format.h>
1166 auto s = fmt::format("{}", 4.2); // formats 4.2 using Grisu
1169 With Grisu enabled, {fmt} is 13x faster than `std::ostringstream`
1170 (libc++) and 10x faster than `sprintf` on
1171 [dtoa-benchmark](https://github.com/fmtlib/dtoa-benchmark) ([full
1172 results](https://fmt.dev/unknown_mac64_clang10.0.html)):
1174 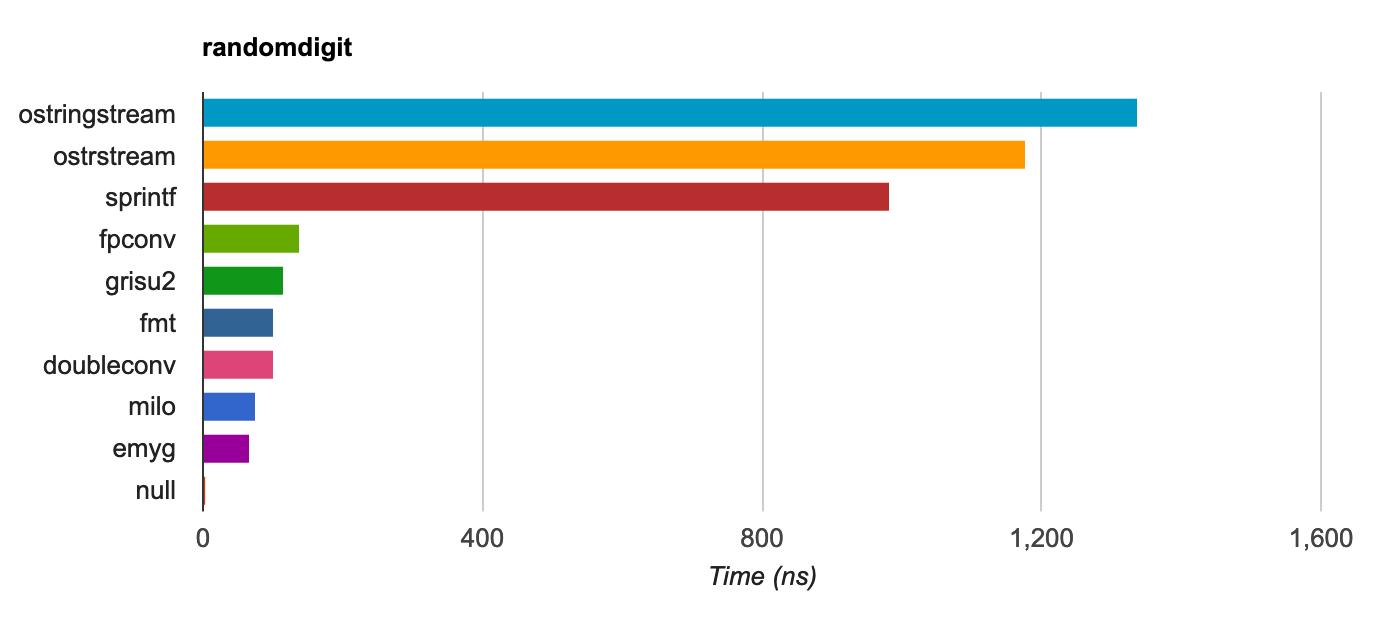
1176 - Separated formatting and parsing contexts for consistency with
1177 [C++20 std::format](http://eel.is/c++draft/format), removing the
1178 undocumented `basic_format_context::parse_context()` function.
1180 - Added [oss-fuzz](https://github.com/google/oss-fuzz) support
1181 (https://github.com/fmtlib/fmt/pull/1199). Thanks @pauldreik.
1183 - `formatter` specializations now always take precedence over
1184 `operator<<` (https://github.com/fmtlib/fmt/issues/952):
1188 #include <fmt/ostream.h>
1192 std::ostream& operator<<(std::ostream& os, S) {
1197 struct fmt::formatter<S> : fmt::formatter<int> {
1198 auto format(S, format_context& ctx) {
1199 return formatter<int>::format(2, ctx);
1204 std::cout << S() << "\n"; // prints 1 using operator<<
1205 fmt::print("{}\n", S()); // prints 2 using formatter
1209 - Introduced the experimental `fmt::compile` function that does format
1211 (https://github.com/fmtlib/fmt/issues/618,
1212 https://github.com/fmtlib/fmt/issues/1169,
1213 https://github.com/fmtlib/fmt/pull/1171):
1216 #include <fmt/compile.h>
1218 auto f = fmt::compile<int>("{}");
1219 std::string s = fmt::format(f, 42); // can be called multiple times to
1220 // format different values
1224 It moves the cost of parsing a format string outside of the format
1225 function which can be beneficial when identically formatting many
1226 objects of the same types. Thanks @stryku.
1228 - Added experimental `%` format specifier that formats floating-point
1229 values as percentages
1230 (https://github.com/fmtlib/fmt/pull/1060,
1231 https://github.com/fmtlib/fmt/pull/1069,
1232 https://github.com/fmtlib/fmt/pull/1071):
1235 auto s = fmt::format("{:.1%}", 0.42); // s == "42.0%"
1238 Thanks @gawain-bolton.
1240 - Implemented precision for floating-point durations
1241 (https://github.com/fmtlib/fmt/issues/1004,
1242 https://github.com/fmtlib/fmt/pull/1012):
1245 auto s = fmt::format("{:.1}", std::chrono::duration<double>(1.234));
1251 - Implemented `chrono` format specifiers `%Q` and `%q` that give the
1252 value and the unit respectively
1253 (https://github.com/fmtlib/fmt/pull/1019):
1256 auto value = fmt::format("{:%Q}", 42s); // value == "42"
1257 auto unit = fmt::format("{:%q}", 42s); // unit == "s"
1262 - Fixed handling of dynamic width in chrono formatter:
1265 auto s = fmt::format("{0:{1}%H:%M:%S}", std::chrono::seconds(12345), 12);
1266 // ^ width argument index ^ width
1270 Thanks Howard Hinnant.
1272 - Removed deprecated `fmt/time.h`. Use `fmt/chrono.h` instead.
1274 - Added `fmt::format` and `fmt::vformat` overloads that take
1275 `text_style` (https://github.com/fmtlib/fmt/issues/993,
1276 https://github.com/fmtlib/fmt/pull/994):
1279 #include <fmt/color.h>
1281 std::string message = fmt::format(fmt::emphasis::bold | fg(fmt::color::red),
1282 "The answer is {}.", 42);
1287 - Removed the deprecated color API (`print_colored`). Use the new API,
1288 namely `print` overloads that take `text_style` instead.
1290 - Made `std::unique_ptr` and `std::shared_ptr` formattable as pointers
1291 via `fmt::ptr` (https://github.com/fmtlib/fmt/pull/1121):
1294 std::unique_ptr<int> p = ...;
1295 fmt::print("{}", fmt::ptr(p)); // prints p as a pointer
1300 - Made `print` and `vprint` report I/O errors
1301 (https://github.com/fmtlib/fmt/issues/1098,
1302 https://github.com/fmtlib/fmt/pull/1099). Thanks @BillyDonahue.
1304 - Marked deprecated APIs with the `[[deprecated]]` attribute and
1305 removed internal uses of deprecated APIs
1306 (https://github.com/fmtlib/fmt/pull/1022). Thanks @eliaskosunen.
1308 - Modernized the codebase using more C++11 features and removing
1309 workarounds. Most importantly, `buffer_context` is now an alias
1310 template, so use `buffer_context<T>` instead of
1311 `buffer_context<T>::type`. These features require GCC 4.8 or later.
1313 - `formatter` specializations now always take precedence over implicit
1314 conversions to `int` and the undocumented `convert_to_int` trait is
1317 - Moved the undocumented `basic_writer`, `writer`, and `wwriter` types
1318 to the `internal` namespace.
1320 - Removed deprecated `basic_format_context::begin()`. Use `out()`
1323 - Disallowed passing the result of `join` as an lvalue to prevent
1326 - Refactored the undocumented structs that represent parsed format
1327 specifiers to simplify the API and allow multibyte fill.
1329 - Moved SFINAE to template parameters to reduce symbol sizes.
1331 - Switched to `fputws` for writing wide strings so that it\'s no
1332 longer required to call `_setmode` on Windows
1333 (https://github.com/fmtlib/fmt/issues/1229,
1334 https://github.com/fmtlib/fmt/pull/1243). Thanks @jackoalan.
1336 - Improved literal-based API
1337 (https://github.com/fmtlib/fmt/pull/1254). Thanks @sylveon.
1339 - Added support for exotic platforms without `uintptr_t` such as IBM i
1340 (AS/400) which has 128-bit pointers and only 64-bit integers
1341 (https://github.com/fmtlib/fmt/issues/1059).
1343 - Added [Sublime Text syntax highlighting config](
1344 https://github.com/fmtlib/fmt/blob/master/support/C%2B%2B.sublime-syntax)
1345 (https://github.com/fmtlib/fmt/issues/1037). Thanks @Kronuz.
1347 - Added the `FMT_ENFORCE_COMPILE_STRING` macro to enforce the use of
1348 compile-time format strings
1349 (https://github.com/fmtlib/fmt/pull/1231). Thanks @jackoalan.
1351 - Stopped setting `CMAKE_BUILD_TYPE` if {fmt} is a subproject
1352 (https://github.com/fmtlib/fmt/issues/1081).
1354 - Various build improvements
1355 (https://github.com/fmtlib/fmt/pull/1039,
1356 https://github.com/fmtlib/fmt/pull/1078,
1357 https://github.com/fmtlib/fmt/pull/1091,
1358 https://github.com/fmtlib/fmt/pull/1103,
1359 https://github.com/fmtlib/fmt/pull/1177).
1360 Thanks @luncliff, @jasonszang, @olafhering, @Lecetem and @pauldreik.
1362 - Improved documentation
1363 (https://github.com/fmtlib/fmt/issues/1049,
1364 https://github.com/fmtlib/fmt/pull/1051,
1365 https://github.com/fmtlib/fmt/pull/1083,
1366 https://github.com/fmtlib/fmt/pull/1113,
1367 https://github.com/fmtlib/fmt/pull/1114,
1368 https://github.com/fmtlib/fmt/issues/1146,
1369 https://github.com/fmtlib/fmt/issues/1180,
1370 https://github.com/fmtlib/fmt/pull/1250,
1371 https://github.com/fmtlib/fmt/pull/1252,
1372 https://github.com/fmtlib/fmt/pull/1265).
1373 Thanks @mikelui, @foonathan, @BillyDonahue, @jwakely, @kaisbe and
1376 - Fixed ambiguous formatter specialization in `fmt/ranges.h`
1377 (https://github.com/fmtlib/fmt/issues/1123).
1379 - Fixed formatting of a non-empty `std::filesystem::path` which is an
1380 infinitely deep range of its components
1381 (https://github.com/fmtlib/fmt/issues/1268).
1383 - Fixed handling of general output iterators when formatting
1384 characters (https://github.com/fmtlib/fmt/issues/1056,
1385 https://github.com/fmtlib/fmt/pull/1058). Thanks @abolz.
1387 - Fixed handling of output iterators in `formatter` specialization for
1388 ranges (https://github.com/fmtlib/fmt/issues/1064).
1390 - Fixed handling of exotic character types
1391 (https://github.com/fmtlib/fmt/issues/1188).
1393 - Made chrono formatting work with exceptions disabled
1394 (https://github.com/fmtlib/fmt/issues/1062).
1396 - Fixed DLL visibility issues
1397 (https://github.com/fmtlib/fmt/pull/1134,
1398 https://github.com/fmtlib/fmt/pull/1147). Thanks @denchat.
1400 - Disabled the use of UDL template extension on GCC 9
1401 (https://github.com/fmtlib/fmt/issues/1148).
1403 - Removed misplaced `format` compile-time checks from `printf`
1404 (https://github.com/fmtlib/fmt/issues/1173).
1406 - Fixed issues in the experimental floating-point formatter
1407 (https://github.com/fmtlib/fmt/issues/1072,
1408 https://github.com/fmtlib/fmt/issues/1129,
1409 https://github.com/fmtlib/fmt/issues/1153,
1410 https://github.com/fmtlib/fmt/pull/1155,
1411 https://github.com/fmtlib/fmt/issues/1210,
1412 https://github.com/fmtlib/fmt/issues/1222). Thanks @alabuzhev.
1414 - Fixed bugs discovered by fuzzing or during fuzzing integration
1415 (https://github.com/fmtlib/fmt/issues/1124,
1416 https://github.com/fmtlib/fmt/issues/1127,
1417 https://github.com/fmtlib/fmt/issues/1132,
1418 https://github.com/fmtlib/fmt/pull/1135,
1419 https://github.com/fmtlib/fmt/issues/1136,
1420 https://github.com/fmtlib/fmt/issues/1141,
1421 https://github.com/fmtlib/fmt/issues/1142,
1422 https://github.com/fmtlib/fmt/issues/1178,
1423 https://github.com/fmtlib/fmt/issues/1179,
1424 https://github.com/fmtlib/fmt/issues/1194). Thanks @pauldreik.
1426 - Fixed building tests on FreeBSD and Hurd
1427 (https://github.com/fmtlib/fmt/issues/1043). Thanks @jackyf.
1429 - Fixed various warnings and compilation issues
1430 (https://github.com/fmtlib/fmt/pull/998,
1431 https://github.com/fmtlib/fmt/pull/1006,
1432 https://github.com/fmtlib/fmt/issues/1008,
1433 https://github.com/fmtlib/fmt/issues/1011,
1434 https://github.com/fmtlib/fmt/issues/1025,
1435 https://github.com/fmtlib/fmt/pull/1027,
1436 https://github.com/fmtlib/fmt/pull/1028,
1437 https://github.com/fmtlib/fmt/pull/1029,
1438 https://github.com/fmtlib/fmt/pull/1030,
1439 https://github.com/fmtlib/fmt/pull/1031,
1440 https://github.com/fmtlib/fmt/pull/1054,
1441 https://github.com/fmtlib/fmt/issues/1063,
1442 https://github.com/fmtlib/fmt/pull/1068,
1443 https://github.com/fmtlib/fmt/pull/1074,
1444 https://github.com/fmtlib/fmt/pull/1075,
1445 https://github.com/fmtlib/fmt/pull/1079,
1446 https://github.com/fmtlib/fmt/pull/1086,
1447 https://github.com/fmtlib/fmt/issues/1088,
1448 https://github.com/fmtlib/fmt/pull/1089,
1449 https://github.com/fmtlib/fmt/pull/1094,
1450 https://github.com/fmtlib/fmt/issues/1101,
1451 https://github.com/fmtlib/fmt/pull/1102,
1452 https://github.com/fmtlib/fmt/issues/1105,
1453 https://github.com/fmtlib/fmt/pull/1107,
1454 https://github.com/fmtlib/fmt/issues/1115,
1455 https://github.com/fmtlib/fmt/issues/1117,
1456 https://github.com/fmtlib/fmt/issues/1118,
1457 https://github.com/fmtlib/fmt/issues/1120,
1458 https://github.com/fmtlib/fmt/issues/1123,
1459 https://github.com/fmtlib/fmt/pull/1139,
1460 https://github.com/fmtlib/fmt/issues/1140,
1461 https://github.com/fmtlib/fmt/issues/1143,
1462 https://github.com/fmtlib/fmt/pull/1144,
1463 https://github.com/fmtlib/fmt/pull/1150,
1464 https://github.com/fmtlib/fmt/pull/1151,
1465 https://github.com/fmtlib/fmt/issues/1152,
1466 https://github.com/fmtlib/fmt/issues/1154,
1467 https://github.com/fmtlib/fmt/issues/1156,
1468 https://github.com/fmtlib/fmt/pull/1159,
1469 https://github.com/fmtlib/fmt/issues/1175,
1470 https://github.com/fmtlib/fmt/issues/1181,
1471 https://github.com/fmtlib/fmt/issues/1186,
1472 https://github.com/fmtlib/fmt/pull/1187,
1473 https://github.com/fmtlib/fmt/pull/1191,
1474 https://github.com/fmtlib/fmt/issues/1197,
1475 https://github.com/fmtlib/fmt/issues/1200,
1476 https://github.com/fmtlib/fmt/issues/1203,
1477 https://github.com/fmtlib/fmt/issues/1205,
1478 https://github.com/fmtlib/fmt/pull/1206,
1479 https://github.com/fmtlib/fmt/issues/1213,
1480 https://github.com/fmtlib/fmt/issues/1214,
1481 https://github.com/fmtlib/fmt/pull/1217,
1482 https://github.com/fmtlib/fmt/issues/1228,
1483 https://github.com/fmtlib/fmt/pull/1230,
1484 https://github.com/fmtlib/fmt/issues/1232,
1485 https://github.com/fmtlib/fmt/pull/1235,
1486 https://github.com/fmtlib/fmt/pull/1236,
1487 https://github.com/fmtlib/fmt/issues/1240).
1488 Thanks @DanielaE, @mwinterb, @eliaskosunen, @morinmorin, @ricco19,
1489 @waywardmonkeys, @chronoxor, @remyabel, @pauldreik, @gsjaardema, @rcane,
1490 @mocabe, @denchat, @cjdb, @HazardyKnusperkeks, @vedranmiletic, @jackoalan,
1491 @DaanDeMeyer and @starkmapper.
1493 # 5.3.0 - 2018-12-28
1495 - Introduced experimental chrono formatting support:
1498 #include <fmt/chrono.h>
1501 using namespace std::literals::chrono_literals;
1502 fmt::print("Default format: {} {}\n", 42s, 100ms);
1503 fmt::print("strftime-like format: {:%H:%M:%S}\n", 3h + 15min + 30s);
1509 Default format: 42s 100ms
1510 strftime-like format: 03:15:30
1512 - Added experimental support for emphasis (bold, italic, underline,
1513 strikethrough), colored output to a file stream, and improved
1514 colored formatting API
1515 (https://github.com/fmtlib/fmt/pull/961,
1516 https://github.com/fmtlib/fmt/pull/967,
1517 https://github.com/fmtlib/fmt/pull/973):
1520 #include <fmt/color.h>
1523 fmt::print(fg(fmt::color::crimson) | fmt::emphasis::bold,
1524 "Hello, {}!\n", "world");
1525 fmt::print(fg(fmt::color::floral_white) | bg(fmt::color::slate_gray) |
1526 fmt::emphasis::underline, "OlĂĄ, {}!\n", "Mundo");
1527 fmt::print(fg(fmt::color::steel_blue) | fmt::emphasis::italic,
1528 "äœ ć„œ{}ïŒ\n", "äžç");
1532 prints the following on modern terminals with RGB color support:
1534 
1538 - Added support for 4-bit terminal colors
1539 (https://github.com/fmtlib/fmt/issues/968,
1540 https://github.com/fmtlib/fmt/pull/974)
1543 #include <fmt/color.h>
1546 print(fg(fmt::terminal_color::red), "stop\n");
1550 Note that these colors vary by terminal:
1552 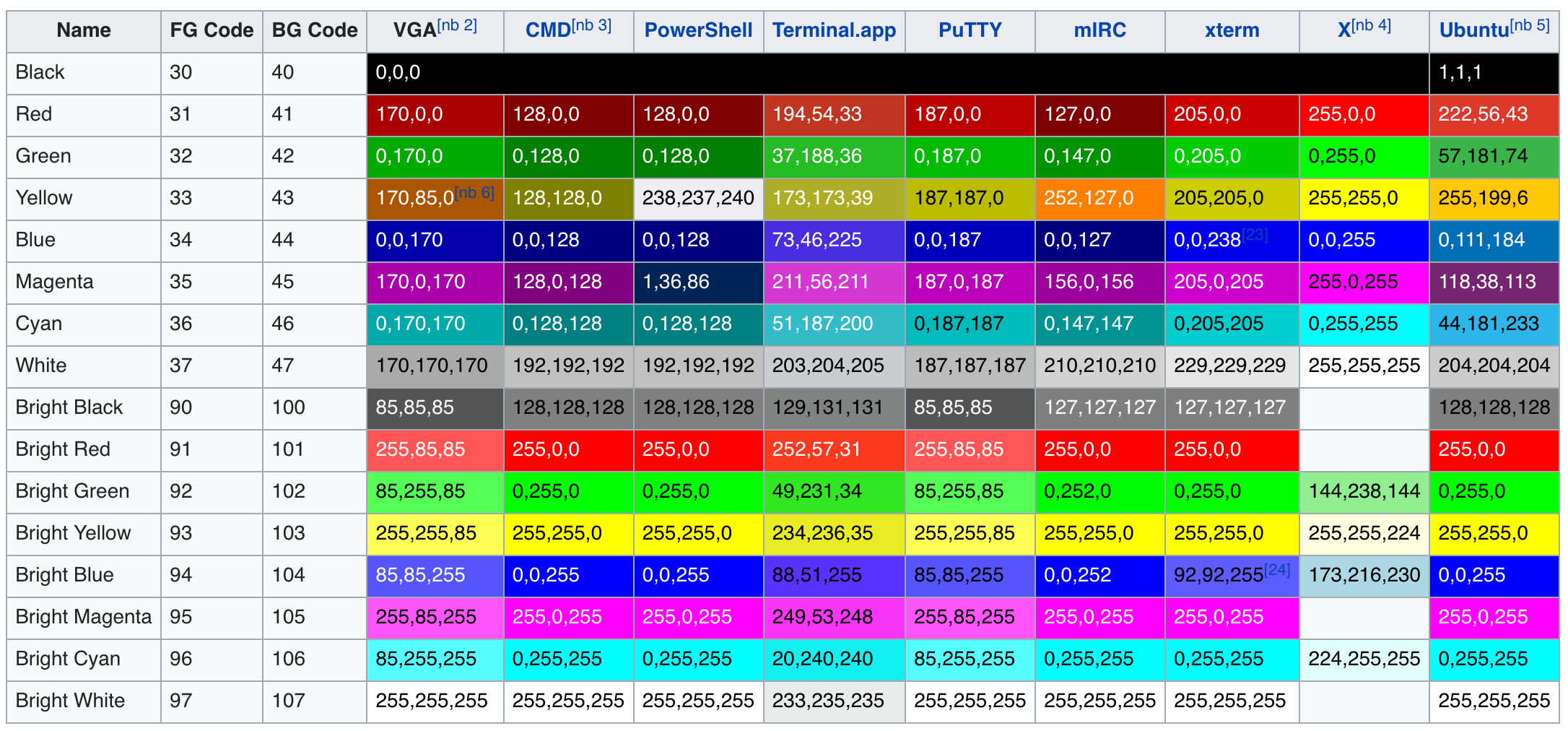
1556 - Parameterized formatting functions on the type of the format string
1557 (https://github.com/fmtlib/fmt/issues/880,
1558 https://github.com/fmtlib/fmt/pull/881,
1559 https://github.com/fmtlib/fmt/pull/883,
1560 https://github.com/fmtlib/fmt/pull/885,
1561 https://github.com/fmtlib/fmt/pull/897,
1562 https://github.com/fmtlib/fmt/issues/920). Any object of
1563 type `S` that has an overloaded `to_string_view(const S&)` returning
1564 `fmt::string_view` can be used as a format string:
1568 inline string_view to_string_view(const my_string& s) {
1569 return {s.data(), s.length()};
1573 std::string message = fmt::format(my_string("The answer is {}."), 42);
1578 - Made `std::string_view` work as a format string
1579 (https://github.com/fmtlib/fmt/pull/898):
1582 auto message = fmt::format(std::string_view("The answer is {}."), 42);
1587 - Added wide string support to compile-time format string checks
1588 (https://github.com/fmtlib/fmt/pull/924):
1591 print(fmt(L"{:f}"), 42); // compile-time error: invalid type specifier
1596 - Made colored print functions work with wide strings
1597 (https://github.com/fmtlib/fmt/pull/867):
1600 #include <fmt/color.h>
1603 print(fg(fmt::color::red), L"{}\n", 42);
1609 - Introduced experimental Unicode support
1610 (https://github.com/fmtlib/fmt/issues/628,
1611 https://github.com/fmtlib/fmt/pull/891):
1614 using namespace fmt::literals;
1615 auto s = fmt::format("{:*^5}"_u, "đ€Ą"_u); // s == "**đ€Ą**"_u
1618 - Improved locale support:
1621 #include <fmt/locale.h>
1623 struct numpunct : std::numpunct<char> {
1625 char do_thousands_sep() const override { return '~'; }
1629 auto s = fmt::format(std::locale(loc, new numpunct()), "{:n}", 1234567);
1633 - Constrained formatting functions on proper iterator types
1634 (https://github.com/fmtlib/fmt/pull/921). Thanks @DanielaE.
1636 - Added `make_printf_args` and `make_wprintf_args` functions
1637 (https://github.com/fmtlib/fmt/pull/934). Thanks @tnovotny.
1639 - Deprecated `fmt::visit`, `parse_context`, and `wparse_context`. Use
1640 `fmt::visit_format_arg`, `format_parse_context`, and
1641 `wformat_parse_context` instead.
1643 - Removed undocumented `basic_fixed_buffer` which has been superseded
1644 by the iterator-based API
1645 (https://github.com/fmtlib/fmt/issues/873,
1646 https://github.com/fmtlib/fmt/pull/902). Thanks @superfunc.
1648 - Disallowed repeated leading zeros in an argument ID:
1651 fmt::print("{000}", 42); // error
1654 - Reintroduced support for gcc 4.4.
1656 - Fixed compilation on platforms with exotic `double`
1657 (https://github.com/fmtlib/fmt/issues/878).
1659 - Improved documentation
1660 (https://github.com/fmtlib/fmt/issues/164,
1661 https://github.com/fmtlib/fmt/issues/877,
1662 https://github.com/fmtlib/fmt/pull/901,
1663 https://github.com/fmtlib/fmt/pull/906,
1664 https://github.com/fmtlib/fmt/pull/979).
1665 Thanks @kookjr, @DarkDimius and @HecticSerenity.
1667 - Added pkgconfig support which makes it easier to consume the library
1668 from meson and other build systems
1669 (https://github.com/fmtlib/fmt/pull/916). Thanks @colemickens.
1671 - Various build improvements
1672 (https://github.com/fmtlib/fmt/pull/909,
1673 https://github.com/fmtlib/fmt/pull/926,
1674 https://github.com/fmtlib/fmt/pull/937,
1675 https://github.com/fmtlib/fmt/pull/953,
1676 https://github.com/fmtlib/fmt/pull/959).
1677 Thanks @tchaikov, @luncliff, @AndreasSchoenle, @hotwatermorning and @Zefz.
1679 - Improved `string_view` construction performance
1680 (https://github.com/fmtlib/fmt/pull/914). Thanks @gabime.
1682 - Fixed non-matching char types
1683 (https://github.com/fmtlib/fmt/pull/895). Thanks @DanielaE.
1685 - Fixed `format_to_n` with `std::back_insert_iterator`
1686 (https://github.com/fmtlib/fmt/pull/913). Thanks @DanielaE.
1688 - Fixed locale-dependent formatting
1689 (https://github.com/fmtlib/fmt/issues/905).
1691 - Fixed various compiler warnings and errors
1692 (https://github.com/fmtlib/fmt/pull/882,
1693 https://github.com/fmtlib/fmt/pull/886,
1694 https://github.com/fmtlib/fmt/pull/933,
1695 https://github.com/fmtlib/fmt/pull/941,
1696 https://github.com/fmtlib/fmt/issues/931,
1697 https://github.com/fmtlib/fmt/pull/943,
1698 https://github.com/fmtlib/fmt/pull/954,
1699 https://github.com/fmtlib/fmt/pull/956,
1700 https://github.com/fmtlib/fmt/pull/962,
1701 https://github.com/fmtlib/fmt/issues/965,
1702 https://github.com/fmtlib/fmt/issues/977,
1703 https://github.com/fmtlib/fmt/pull/983,
1704 https://github.com/fmtlib/fmt/pull/989).
1705 Thanks @Luthaf, @stevenhoving, @christinaa, @lgritz, @DanielaE,
1706 @0x8000-0000 and @liuping1997.
1708 # 5.2.1 - 2018-09-21
1710 - Fixed `visit` lookup issues on gcc 7 & 8
1711 (https://github.com/fmtlib/fmt/pull/870). Thanks @medithe.
1712 - Fixed linkage errors on older gcc.
1713 - Prevented `fmt/range.h` from specializing `fmt::basic_string_view`
1714 (https://github.com/fmtlib/fmt/issues/865,
1715 https://github.com/fmtlib/fmt/pull/868). Thanks @hhggit.
1716 - Improved error message when formatting unknown types
1717 (https://github.com/fmtlib/fmt/pull/872). Thanks @foonathan.
1718 - Disabled templated user-defined literals when compiled under nvcc
1719 (https://github.com/fmtlib/fmt/pull/875). Thanks @CandyGumdrop.
1720 - Fixed `format_to` formatting to `wmemory_buffer`
1721 (https://github.com/fmtlib/fmt/issues/874).
1723 # 5.2.0 - 2018-09-13
1725 - Optimized format string parsing and argument processing which
1726 resulted in up to 5x speed up on long format strings and significant
1727 performance boost on various benchmarks. For example, version 5.2 is
1728 2.22x faster than 5.1 on decimal integer formatting with `format_to`
1729 (macOS, clang-902.0.39.2):
1731 | Method | Time, s | Speedup |
1732 | -------------------------- | --------------: | ------: |
1733 | fmt::format 5.1 | 0.58 | |
1734 | fmt::format 5.2 | 0.35 | 1.66x |
1735 | fmt::format_to 5.1 | 0.51 | |
1736 | fmt::format_to 5.2 | 0.23 | 2.22x |
1737 | sprintf | 0.71 | |
1738 | std::to_string | 1.01 | |
1739 | std::stringstream | 1.73 | |
1741 - Changed the `fmt` macro from opt-out to opt-in to prevent name
1742 collisions. To enable it define the `FMT_STRING_ALIAS` macro to 1
1743 before including `fmt/format.h`:
1746 #define FMT_STRING_ALIAS 1
1747 #include <fmt/format.h>
1748 std::string answer = format(fmt("{}"), 42);
1751 - Added compile-time format string checks to `format_to` overload that
1752 takes `fmt::memory_buffer`
1753 (https://github.com/fmtlib/fmt/issues/783):
1756 fmt::memory_buffer buf;
1757 // Compile-time error: invalid type specifier.
1758 fmt::format_to(buf, fmt("{:d}"), "foo");
1761 - Moved experimental color support to `fmt/color.h` and enabled the
1762 new API by default. The old API can be enabled by defining the
1763 `FMT_DEPRECATED_COLORS` macro.
1765 - Added formatting support for types explicitly convertible to
1770 explicit operator fmt::string_view() const { return "foo"; }
1772 auto s = format("{}", foo());
1775 In particular, this makes formatting function work with
1776 `folly::StringPiece`.
1778 - Implemented preliminary support for `char*_t` by replacing the
1779 `format` function overloads with a single function template
1780 parameterized on the string type.
1782 - Added support for dynamic argument lists
1783 (https://github.com/fmtlib/fmt/issues/814,
1784 https://github.com/fmtlib/fmt/pull/819). Thanks @MikePopoloski.
1786 - Reduced executable size overhead for embedded targets using newlib
1787 nano by making locale dependency optional
1788 (https://github.com/fmtlib/fmt/pull/839). Thanks @teajay-fr.
1790 - Keep `noexcept` specifier when exceptions are disabled
1791 (https://github.com/fmtlib/fmt/issues/801,
1792 https://github.com/fmtlib/fmt/pull/810). Thanks @qis.
1794 - Fixed formatting of user-defined types providing `operator<<` with
1795 `format_to_n` (https://github.com/fmtlib/fmt/pull/806).
1798 - Fixed dynamic linkage of new symbols
1799 (https://github.com/fmtlib/fmt/issues/808).
1801 - Fixed global initialization issue
1802 (https://github.com/fmtlib/fmt/issues/807):
1805 // This works on compilers with constexpr support.
1806 static const std::string answer = fmt::format("{}", 42);
1809 - Fixed various compiler warnings and errors
1810 (https://github.com/fmtlib/fmt/pull/804,
1811 https://github.com/fmtlib/fmt/issues/809,
1812 https://github.com/fmtlib/fmt/pull/811,
1813 https://github.com/fmtlib/fmt/issues/822,
1814 https://github.com/fmtlib/fmt/pull/827,
1815 https://github.com/fmtlib/fmt/issues/830,
1816 https://github.com/fmtlib/fmt/pull/838,
1817 https://github.com/fmtlib/fmt/issues/843,
1818 https://github.com/fmtlib/fmt/pull/844,
1819 https://github.com/fmtlib/fmt/issues/851,
1820 https://github.com/fmtlib/fmt/pull/852,
1821 https://github.com/fmtlib/fmt/pull/854).
1822 Thanks @henryiii, @medithe, and @eliasdaler.
1824 # 5.1.0 - 2018-07-05
1826 - Added experimental support for RGB color output enabled with the
1827 `FMT_EXTENDED_COLORS` macro:
1830 #define FMT_EXTENDED_COLORS
1831 #define FMT_HEADER_ONLY // or compile fmt with FMT_EXTENDED_COLORS defined
1832 #include <fmt/format.h>
1834 fmt::print(fmt::color::steel_blue, "Some beautiful text");
1837 The old API (the `print_colored` and `vprint_colored` functions and
1838 the `color` enum) is now deprecated.
1839 (https://github.com/fmtlib/fmt/issues/762
1840 https://github.com/fmtlib/fmt/pull/767). thanks @Remotion.
1842 - Added quotes to strings in ranges and tuples
1843 (https://github.com/fmtlib/fmt/pull/766). Thanks @Remotion.
1845 - Made `format_to` work with `basic_memory_buffer`
1846 (https://github.com/fmtlib/fmt/issues/776).
1848 - Added `vformat_to_n` and `wchar_t` overload of `format_to_n`
1849 (https://github.com/fmtlib/fmt/issues/764,
1850 https://github.com/fmtlib/fmt/issues/769).
1852 - Made `is_range` and `is_tuple_like` part of public (experimental)
1853 API to allow specialization for user-defined types
1854 (https://github.com/fmtlib/fmt/issues/751,
1855 https://github.com/fmtlib/fmt/pull/759). Thanks @drrlvn.
1857 - Added more compilers to continuous integration and increased
1858 `FMT_PEDANTIC` warning levels
1859 (https://github.com/fmtlib/fmt/pull/736). Thanks @eliaskosunen.
1861 - Fixed compilation with MSVC 2013.
1863 - Fixed handling of user-defined types in `format_to`
1864 (https://github.com/fmtlib/fmt/issues/793).
1866 - Forced linking of inline `vformat` functions into the library
1867 (https://github.com/fmtlib/fmt/issues/795).
1869 - Fixed incorrect call to on_align in `'{:}='`
1870 (https://github.com/fmtlib/fmt/issues/750).
1872 - Fixed floating-point formatting to a non-back_insert_iterator with
1873 sign & numeric alignment specified
1874 (https://github.com/fmtlib/fmt/issues/756).
1876 - Fixed formatting to an array with `format_to_n`
1877 (https://github.com/fmtlib/fmt/issues/778).
1879 - Fixed formatting of more than 15 named arguments
1880 (https://github.com/fmtlib/fmt/issues/754).
1882 - Fixed handling of compile-time strings when including
1883 `fmt/ostream.h`. (https://github.com/fmtlib/fmt/issues/768).
1885 - Fixed various compiler warnings and errors
1886 (https://github.com/fmtlib/fmt/issues/742,
1887 https://github.com/fmtlib/fmt/issues/748,
1888 https://github.com/fmtlib/fmt/issues/752,
1889 https://github.com/fmtlib/fmt/issues/770,
1890 https://github.com/fmtlib/fmt/pull/775,
1891 https://github.com/fmtlib/fmt/issues/779,
1892 https://github.com/fmtlib/fmt/pull/780,
1893 https://github.com/fmtlib/fmt/pull/790,
1894 https://github.com/fmtlib/fmt/pull/792,
1895 https://github.com/fmtlib/fmt/pull/800).
1896 Thanks @Remotion, @gabime, @foonathan, @Dark-Passenger and @0x8000-0000.
1898 # 5.0.0 - 2018-05-21
1900 - Added a requirement for partial C++11 support, most importantly
1901 variadic templates and type traits, and dropped `FMT_VARIADIC_*`
1902 emulation macros. Variadic templates are available since GCC 4.4,
1903 Clang 2.9 and MSVC 18.0 (2013). For older compilers use {fmt}
1904 [version 4.x](https://github.com/fmtlib/fmt/releases/tag/4.1.0)
1905 which continues to be maintained and works with C++98 compilers.
1907 - Renamed symbols to follow standard C++ naming conventions and
1908 proposed a subset of the library for standardization in [P0645R2
1909 Text Formatting](https://wg21.link/P0645).
1911 - Implemented `constexpr` parsing of format strings and [compile-time
1913 checks](https://fmt.dev/latest/api.html#compile-time-format-string-checks).
1917 #include <fmt/format.h>
1919 std::string s = format(fmt("{:d}"), "foo");
1922 gives a compile-time error because `d` is an invalid specifier for
1923 strings ([godbolt](https://godbolt.org/g/rnCy9Q)):
1926 <source>:4:19: note: in instantiation of function template specialization 'fmt::v5::format<S, char [4]>' requested here
1927 std::string s = format(fmt("{:d}"), "foo");
1929 format.h:1337:13: note: non-constexpr function 'on_error' cannot be used in a constant expression
1930 handler.on_error("invalid type specifier");
1932 Compile-time checks require relaxed `constexpr` (C++14 feature)
1933 support. If the latter is not available, checks will be performed at
1936 - Separated format string parsing and formatting in the extension API
1937 to enable compile-time format string processing. For example
1944 struct formatter<Answer> {
1945 constexpr auto parse(parse_context& ctx) {
1946 auto it = ctx.begin();
1948 if (spec != 'd' && spec != 's')
1949 throw format_error("invalid specifier");
1953 template <typename FormatContext>
1954 auto format(Answer, FormatContext& ctx) {
1955 return spec == 's' ?
1956 format_to(ctx.begin(), "{}", "fourty-two") :
1957 format_to(ctx.begin(), "{}", 42);
1964 std::string s = format(fmt("{:x}"), Answer());
1967 gives a compile-time error due to invalid format specifier
1968 ([godbolt](https://godbolt.org/g/2jQ1Dv)):
1971 <source>:12:45: error: expression '<throw-expression>' is not a constant expression
1972 throw format_error("invalid specifier");
1975 support](https://fmt.dev/latest/api.html#output-iterator-support):
1979 #include <fmt/format.h>
1981 std::vector<char> out;
1982 fmt::format_to(std::back_inserter(out), "{}", 42);
1986 [format_to_n](https://fmt.dev/latest/api.html#_CPPv2N3fmt11format_to_nE8OutputItNSt6size_tE11string_viewDpRK4Args)
1987 function that restricts the output to the specified number of
1988 characters (https://github.com/fmtlib/fmt/issues/298):
1992 fmt::format_to_n(out, sizeof(out), "{}", 12345);
1993 // out == "1234" (without terminating '\0')
1996 - Added the [formatted_size](
1997 https://fmt.dev/latest/api.html#_CPPv2N3fmt14formatted_sizeE11string_viewDpRK4Args)
1998 function for computing the output size:
2001 #include <fmt/format.h>
2003 auto size = fmt::formatted_size("{}", 12345); // size == 5
2006 - Improved compile times by reducing dependencies on standard headers
2007 and providing a lightweight [core
2008 API](https://fmt.dev/latest/api.html#core-api):
2011 #include <fmt/core.h>
2013 fmt::print("The answer is {}.", 42);
2016 See [Compile time and code
2017 bloat](https://github.com/fmtlib/fmt#compile-time-and-code-bloat).
2019 - Added the [make_format_args](
2020 https://fmt.dev/latest/api.html#_CPPv2N3fmt16make_format_argsEDpRK4Args)
2021 function for capturing formatting arguments:
2024 // Prints formatted error message.
2025 void vreport_error(const char *format, fmt::format_args args) {
2026 fmt::print("Error: ");
2027 fmt::vprint(format, args);
2029 template <typename... Args>
2030 void report_error(const char *format, const Args & ... args) {
2031 vreport_error(format, fmt::make_format_args(args...));
2035 - Added the `make_printf_args` function for capturing `printf`
2036 arguments (https://github.com/fmtlib/fmt/issues/687,
2037 https://github.com/fmtlib/fmt/pull/694). Thanks @Kronuz.
2039 - Added prefix `v` to non-variadic functions taking `format_args` to
2040 distinguish them from variadic ones:
2043 std::string vformat(string_view format_str, format_args args);
2045 template <typename... Args>
2046 std::string format(string_view format_str, const Args & ... args);
2049 - Added experimental support for formatting ranges, containers and
2050 tuple-like types in `fmt/ranges.h`
2051 (https://github.com/fmtlib/fmt/pull/735):
2054 #include <fmt/ranges.h>
2056 std::vector<int> v = {1, 2, 3};
2057 fmt::print("{}", v); // prints {1, 2, 3}
2062 - Implemented `wchar_t` date and time formatting
2063 (https://github.com/fmtlib/fmt/pull/712):
2066 #include <fmt/time.h>
2068 std::time_t t = std::time(nullptr);
2069 auto s = fmt::format(L"The date is {:%Y-%m-%d}.", *std::localtime(&t));
2074 - Provided more wide string overloads
2075 (https://github.com/fmtlib/fmt/pull/724). Thanks @DanielaE.
2077 - Switched from a custom null-terminated string view class to
2078 `string_view` in the format API and provided `fmt::string_view`
2079 which implements a subset of `std::string_view` API for pre-C++17
2082 - Added support for `std::experimental::string_view`
2083 (https://github.com/fmtlib/fmt/pull/607):
2086 #include <fmt/core.h>
2087 #include <experimental/string_view>
2089 fmt::print("{}", std::experimental::string_view("foo"));
2092 Thanks @virgiliofornazin.
2094 - Allowed mixing named and automatic arguments:
2097 fmt::format("{} {two}", 1, fmt::arg("two", 2));
2100 - Removed the write API in favor of the [format
2101 API](https://fmt.dev/latest/api.html#format-api) with compile-time
2102 handling of format strings.
2104 - Disallowed formatting of multibyte strings into a wide character
2105 target (https://github.com/fmtlib/fmt/pull/606).
2107 - Improved documentation
2108 (https://github.com/fmtlib/fmt/pull/515,
2109 https://github.com/fmtlib/fmt/issues/614,
2110 https://github.com/fmtlib/fmt/pull/617,
2111 https://github.com/fmtlib/fmt/pull/661,
2112 https://github.com/fmtlib/fmt/pull/680).
2113 Thanks @ibell, @mihaitodor and @johnthagen.
2115 - Implemented more efficient handling of large number of format
2118 - Introduced an inline namespace for symbol versioning.
2120 - Added debug postfix `d` to the `fmt` library name
2121 (https://github.com/fmtlib/fmt/issues/636).
2123 - Removed unnecessary `fmt/` prefix in includes
2124 (https://github.com/fmtlib/fmt/pull/397). Thanks @chronoxor.
2126 - Moved `fmt/*.h` to `include/fmt/*.h` to prevent irrelevant files and
2127 directories appearing on the include search paths when fmt is used
2128 as a subproject and moved source files to the `src` directory.
2130 - Added qmake project file `support/fmt.pro`
2131 (https://github.com/fmtlib/fmt/pull/641). Thanks @cowo78.
2133 - Added Gradle build file `support/build.gradle`
2134 (https://github.com/fmtlib/fmt/pull/649). Thanks @luncliff.
2136 - Removed `FMT_CPPFORMAT` CMake option.
2138 - Fixed a name conflict with the macro `CHAR_WIDTH` in glibc
2139 (https://github.com/fmtlib/fmt/pull/616). Thanks @aroig.
2141 - Fixed handling of nested braces in `fmt::join`
2142 (https://github.com/fmtlib/fmt/issues/638).
2144 - Added `SOURCELINK_SUFFIX` for compatibility with Sphinx 1.5
2145 (https://github.com/fmtlib/fmt/pull/497). Thanks @ginggs.
2147 - Added a missing `inline` in the header-only mode
2148 (https://github.com/fmtlib/fmt/pull/626). Thanks @aroig.
2150 - Fixed various compiler warnings
2151 (https://github.com/fmtlib/fmt/pull/640,
2152 https://github.com/fmtlib/fmt/pull/656,
2153 https://github.com/fmtlib/fmt/pull/679,
2154 https://github.com/fmtlib/fmt/pull/681,
2155 https://github.com/fmtlib/fmt/pull/705,
2156 https://github.com/fmtlib/fmt/issues/715,
2157 https://github.com/fmtlib/fmt/pull/717,
2158 https://github.com/fmtlib/fmt/pull/720,
2159 https://github.com/fmtlib/fmt/pull/723,
2160 https://github.com/fmtlib/fmt/pull/726,
2161 https://github.com/fmtlib/fmt/pull/730,
2162 https://github.com/fmtlib/fmt/pull/739).
2163 Thanks @peterbell10, @LarsGullik, @foonathan, @eliaskosunen,
2164 @christianparpart, @DanielaE and @mwinterb.
2166 - Worked around an MSVC bug and fixed several warnings
2167 (https://github.com/fmtlib/fmt/pull/653). Thanks @alabuzhev.
2169 - Worked around GCC bug 67371
2170 (https://github.com/fmtlib/fmt/issues/682).
2172 - Fixed compilation with `-fno-exceptions`
2173 (https://github.com/fmtlib/fmt/pull/655). Thanks @chenxiaolong.
2175 - Made `constexpr remove_prefix` gcc version check tighter
2176 (https://github.com/fmtlib/fmt/issues/648).
2178 - Renamed internal type enum constants to prevent collision with
2179 poorly written C libraries
2180 (https://github.com/fmtlib/fmt/issues/644).
2182 - Added detection of `wostream operator<<`
2183 (https://github.com/fmtlib/fmt/issues/650).
2185 - Fixed compilation on OpenBSD
2186 (https://github.com/fmtlib/fmt/pull/660). Thanks @hubslave.
2188 - Fixed compilation on FreeBSD 12
2189 (https://github.com/fmtlib/fmt/pull/732). Thanks @dankm.
2191 - Fixed compilation when there is a mismatch between `-std` options
2192 between the library and user code
2193 (https://github.com/fmtlib/fmt/issues/664).
2195 - Fixed compilation with GCC 7 and `-std=c++11`
2196 (https://github.com/fmtlib/fmt/issues/734).
2198 - Improved generated binary code on GCC 7 and older
2199 (https://github.com/fmtlib/fmt/issues/668).
2201 - Fixed handling of numeric alignment with no width
2202 (https://github.com/fmtlib/fmt/issues/675).
2204 - Fixed handling of empty strings in UTF8/16 converters
2205 (https://github.com/fmtlib/fmt/pull/676). Thanks @vgalka-sl.
2207 - Fixed formatting of an empty `string_view`
2208 (https://github.com/fmtlib/fmt/issues/689).
2210 - Fixed detection of `string_view` on libc++
2211 (https://github.com/fmtlib/fmt/issues/686).
2213 - Fixed DLL issues (https://github.com/fmtlib/fmt/pull/696).
2216 - Fixed compile checks for mixing narrow and wide strings
2217 (https://github.com/fmtlib/fmt/issues/690).
2219 - Disabled unsafe implicit conversion to `std::string`
2220 (https://github.com/fmtlib/fmt/issues/729).
2222 - Fixed handling of reused format specs (as in `fmt::join`) for
2223 pointers (https://github.com/fmtlib/fmt/pull/725). Thanks @mwinterb.
2225 - Fixed installation of `fmt/ranges.h`
2226 (https://github.com/fmtlib/fmt/pull/738). Thanks @sv1990.
2228 # 4.1.0 - 2017-12-20
2230 - Added `fmt::to_wstring()` in addition to `fmt::to_string()`
2231 (https://github.com/fmtlib/fmt/pull/559). Thanks @alabuzhev.
2232 - Added support for C++17 `std::string_view`
2233 (https://github.com/fmtlib/fmt/pull/571 and
2234 https://github.com/fmtlib/fmt/pull/578).
2235 Thanks @thelostt and @mwinterb.
2236 - Enabled stream exceptions to catch errors
2237 (https://github.com/fmtlib/fmt/issues/581). Thanks @crusader-mike.
2238 - Allowed formatting of class hierarchies with `fmt::format_arg()`
2239 (https://github.com/fmtlib/fmt/pull/547). Thanks @rollbear.
2240 - Removed limitations on character types
2241 (https://github.com/fmtlib/fmt/pull/563). Thanks @Yelnats321.
2242 - Conditionally enabled use of `std::allocator_traits`
2243 (https://github.com/fmtlib/fmt/pull/583). Thanks @mwinterb.
2244 - Added support for `const` variadic member function emulation with
2245 `FMT_VARIADIC_CONST`
2246 (https://github.com/fmtlib/fmt/pull/591). Thanks @ludekvodicka.
2247 - Various bugfixes: bad overflow check, unsupported implicit type
2248 conversion when determining formatting function, test segfaults
2249 (https://github.com/fmtlib/fmt/issues/551), ill-formed
2250 macros (https://github.com/fmtlib/fmt/pull/542) and
2252 (https://github.com/fmtlib/fmt/issues/580). Thanks @xylosper.
2253 - Prevented warnings on MSVC
2254 (https://github.com/fmtlib/fmt/pull/605,
2255 https://github.com/fmtlib/fmt/pull/602, and
2256 https://github.com/fmtlib/fmt/pull/545), clang
2257 (https://github.com/fmtlib/fmt/pull/582), GCC
2258 (https://github.com/fmtlib/fmt/issues/573), various
2259 conversion warnings (https://github.com/fmtlib/fmt/pull/609,
2260 https://github.com/fmtlib/fmt/pull/567,
2261 https://github.com/fmtlib/fmt/pull/553 and
2262 https://github.com/fmtlib/fmt/pull/553), and added
2263 `override` and `[[noreturn]]`
2264 (https://github.com/fmtlib/fmt/pull/549 and
2265 https://github.com/fmtlib/fmt/issues/555).
2266 Thanks @alabuzhev, @virgiliofornazin, @alexanderbock, @yumetodo, @VaderY,
2267 @jpcima, @thelostt and @Manu343726.
2268 - Improved CMake: Used `GNUInstallDirs` to set installation location
2269 (https://github.com/fmtlib/fmt/pull/610) and fixed warnings
2270 (https://github.com/fmtlib/fmt/pull/536 and
2271 https://github.com/fmtlib/fmt/pull/556).
2272 Thanks @mikecrowe, @evgen231 and @henryiii.
2274 # 4.0.0 - 2017-06-27
2276 - Removed old compatibility headers `cppformat/*.h` and CMake options
2277 (https://github.com/fmtlib/fmt/pull/527). Thanks @maddinat0r.
2279 - Added `string.h` containing `fmt::to_string()` as alternative to
2280 `std::to_string()` as well as other string writer functionality
2281 (https://github.com/fmtlib/fmt/issues/326 and
2282 https://github.com/fmtlib/fmt/pull/441):
2285 #include "fmt/string.h"
2287 std::string answer = fmt::to_string(42);
2290 Thanks @glebov-andrey.
2292 - Moved `fmt::printf()` to new `printf.h` header and allowed `%s` as
2293 generic specifier (https://github.com/fmtlib/fmt/pull/453),
2294 made `%.f` more conformant to regular `printf()`
2295 (https://github.com/fmtlib/fmt/pull/490), added custom
2296 writer support (https://github.com/fmtlib/fmt/issues/476)
2297 and implemented missing custom argument formatting
2298 (https://github.com/fmtlib/fmt/pull/339 and
2299 https://github.com/fmtlib/fmt/pull/340):
2302 #include "fmt/printf.h"
2304 // %s format specifier can be used with any argument type.
2305 fmt::printf("%s", 42);
2308 Thanks @mojoBrendan, @manylegged and @spacemoose.
2309 See also https://github.com/fmtlib/fmt/issues/360,
2310 https://github.com/fmtlib/fmt/issues/335 and
2311 https://github.com/fmtlib/fmt/issues/331.
2313 - Added `container.h` containing a `BasicContainerWriter` to write to
2314 containers like `std::vector`
2315 (https://github.com/fmtlib/fmt/pull/450). Thanks @polyvertex.
2317 - Added `fmt::join()` function that takes a range and formats its
2318 elements separated by a given string
2319 (https://github.com/fmtlib/fmt/pull/466):
2322 #include "fmt/format.h"
2324 std::vector<double> v = {1.2, 3.4, 5.6};
2325 // Prints "(+01.20, +03.40, +05.60)".
2326 fmt::print("({:+06.2f})", fmt::join(v.begin(), v.end(), ", "));
2331 - Added support for custom formatting specifications to simplify
2332 customization of built-in formatting
2333 (https://github.com/fmtlib/fmt/pull/444). Thanks @polyvertex.
2334 See also https://github.com/fmtlib/fmt/issues/439.
2336 - Added `fmt::format_system_error()` for error code formatting
2337 (https://github.com/fmtlib/fmt/issues/323 and
2338 https://github.com/fmtlib/fmt/pull/526). Thanks @maddinat0r.
2340 - Added thread-safe `fmt::localtime()` and `fmt::gmtime()` as
2341 replacement for the standard version to `time.h`
2342 (https://github.com/fmtlib/fmt/pull/396). Thanks @codicodi.
2344 - Internal improvements to `NamedArg` and `ArgLists`
2345 (https://github.com/fmtlib/fmt/pull/389 and
2346 https://github.com/fmtlib/fmt/pull/390). Thanks @chronoxor.
2348 - Fixed crash due to bug in `FormatBuf`
2349 (https://github.com/fmtlib/fmt/pull/493). Thanks @effzeh. See also
2350 https://github.com/fmtlib/fmt/issues/480 and
2351 https://github.com/fmtlib/fmt/issues/491.
2353 - Fixed handling of wide strings in `fmt::StringWriter`.
2355 - Improved compiler error messages
2356 (https://github.com/fmtlib/fmt/issues/357).
2358 - Fixed various warnings and issues with various compilers
2359 (https://github.com/fmtlib/fmt/pull/494,
2360 https://github.com/fmtlib/fmt/pull/499,
2361 https://github.com/fmtlib/fmt/pull/483,
2362 https://github.com/fmtlib/fmt/pull/485,
2363 https://github.com/fmtlib/fmt/pull/482,
2364 https://github.com/fmtlib/fmt/pull/475,
2365 https://github.com/fmtlib/fmt/pull/473 and
2366 https://github.com/fmtlib/fmt/pull/414).
2367 Thanks @chronoxor, @zhaohuaxishi, @pkestene, @dschmidt and @0x414c.
2369 - Improved CMake: targets are now namespaced
2370 (https://github.com/fmtlib/fmt/pull/511 and
2371 https://github.com/fmtlib/fmt/pull/513), supported
2372 header-only `printf.h`
2373 (https://github.com/fmtlib/fmt/pull/354), fixed issue with
2374 minimal supported library subset
2375 (https://github.com/fmtlib/fmt/issues/418,
2376 https://github.com/fmtlib/fmt/pull/419 and
2377 https://github.com/fmtlib/fmt/pull/420).
2378 Thanks @bjoernthiel, @niosHD, @LogicalKnight and @alabuzhev.
2380 - Improved documentation (https://github.com/fmtlib/fmt/pull/393).
2383 # 3.0.2 - 2017-06-14
2385 - Added `FMT_VERSION` macro
2386 (https://github.com/fmtlib/fmt/issues/411).
2387 - Used `FMT_NULL` instead of literal `0`
2388 (https://github.com/fmtlib/fmt/pull/409). Thanks @alabuzhev.
2389 - Added extern templates for `format_float`
2390 (https://github.com/fmtlib/fmt/issues/413).
2391 - Fixed implicit conversion issue
2392 (https://github.com/fmtlib/fmt/issues/507).
2393 - Fixed signbit detection
2394 (https://github.com/fmtlib/fmt/issues/423).
2395 - Fixed naming collision
2396 (https://github.com/fmtlib/fmt/issues/425).
2397 - Fixed missing intrinsic for C++/CLI
2398 (https://github.com/fmtlib/fmt/pull/457). Thanks @calumr.
2399 - Fixed Android detection
2400 (https://github.com/fmtlib/fmt/pull/458). Thanks @Gachapen.
2401 - Use lean `windows.h` if not in header-only mode
2402 (https://github.com/fmtlib/fmt/pull/503). Thanks @Quentin01.
2403 - Fixed issue with CMake exporting C++11 flag
2404 (https://github.com/fmtlib/fmt/pull/455). Thanks @EricWF.
2405 - Fixed issue with nvcc and MSVC compiler bug and MinGW
2406 (https://github.com/fmtlib/fmt/issues/505).
2407 - Fixed DLL issues (https://github.com/fmtlib/fmt/pull/469 and
2408 https://github.com/fmtlib/fmt/pull/502).
2409 Thanks @richardeakin and @AndreasSchoenle.
2410 - Fixed test compilation under FreeBSD
2411 (https://github.com/fmtlib/fmt/issues/433).
2412 - Fixed various warnings
2413 (https://github.com/fmtlib/fmt/pull/403,
2414 https://github.com/fmtlib/fmt/pull/410 and
2415 https://github.com/fmtlib/fmt/pull/510).
2416 Thanks @Lecetem, @chenhayat and @trozen.
2417 - Worked around a broken `__builtin_clz` in clang with MS codegen
2418 (https://github.com/fmtlib/fmt/issues/519).
2419 - Removed redundant include
2420 (https://github.com/fmtlib/fmt/issues/479).
2421 - Fixed documentation issues.
2423 # 3.0.1 - 2016-11-01
2425 - Fixed handling of thousands separator
2426 (https://github.com/fmtlib/fmt/issues/353).
2427 - Fixed handling of `unsigned char` strings
2428 (https://github.com/fmtlib/fmt/issues/373).
2429 - Corrected buffer growth when formatting time
2430 (https://github.com/fmtlib/fmt/issues/367).
2431 - Removed warnings under MSVC and clang
2432 (https://github.com/fmtlib/fmt/issues/318,
2433 https://github.com/fmtlib/fmt/issues/250, also merged
2434 https://github.com/fmtlib/fmt/pull/385 and
2435 https://github.com/fmtlib/fmt/pull/361).
2436 Thanks @jcelerier and @nmoehrle.
2437 - Fixed compilation issues under Android
2438 (https://github.com/fmtlib/fmt/pull/327,
2439 https://github.com/fmtlib/fmt/issues/345 and
2440 https://github.com/fmtlib/fmt/pull/381), FreeBSD
2441 (https://github.com/fmtlib/fmt/pull/358), Cygwin
2442 (https://github.com/fmtlib/fmt/issues/388), MinGW
2443 (https://github.com/fmtlib/fmt/issues/355) as well as other
2444 issues (https://github.com/fmtlib/fmt/issues/350,
2445 https://github.com/fmtlib/fmt/issues/355,
2446 https://github.com/fmtlib/fmt/pull/348,
2447 https://github.com/fmtlib/fmt/pull/402,
2448 https://github.com/fmtlib/fmt/pull/405).
2449 Thanks @dpantele, @hghwng, @arvedarved, @LogicalKnight and @JanHellwig.
2450 - Fixed some documentation issues and extended specification
2451 (https://github.com/fmtlib/fmt/issues/320,
2452 https://github.com/fmtlib/fmt/pull/333,
2453 https://github.com/fmtlib/fmt/issues/347,
2454 https://github.com/fmtlib/fmt/pull/362). Thanks @smellman.
2456 # 3.0.0 - 2016-05-07
2458 - The project has been renamed from C++ Format (cppformat) to fmt for
2459 consistency with the used namespace and macro prefix
2460 (https://github.com/fmtlib/fmt/issues/307). Library headers
2461 are now located in the `fmt` directory:
2464 #include "fmt/format.h"
2467 Including `format.h` from the `cppformat` directory is deprecated
2468 but works via a proxy header which will be removed in the next major
2471 The documentation is now available at <https://fmt.dev>.
2474 [strftime](http://en.cppreference.com/w/cpp/chrono/c/strftime)-like
2476 formatting](https://fmt.dev/3.0.0/api.html#date-and-time-formatting)
2477 (https://github.com/fmtlib/fmt/issues/283):
2480 #include "fmt/time.h"
2482 std::time_t t = std::time(nullptr);
2483 // Prints "The date is 2016-04-29." (with the current date)
2484 fmt::print("The date is {:%Y-%m-%d}.", *std::localtime(&t));
2487 - `std::ostream` support including formatting of user-defined types
2488 that provide overloaded `operator<<` has been moved to
2492 #include "fmt/ostream.h"
2495 int year_, month_, day_;
2497 Date(int year, int month, int day) : year_(year), month_(month), day_(day) {}
2499 friend std::ostream &operator<<(std::ostream &os, const Date &d) {
2500 return os << d.year_ << '-' << d.month_ << '-' << d.day_;
2504 std::string s = fmt::format("The date is {}", Date(2012, 12, 9));
2505 // s == "The date is 2012-12-9"
2508 - Added support for [custom argument
2509 formatters](https://fmt.dev/3.0.0/api.html#argument-formatters)
2510 (https://github.com/fmtlib/fmt/issues/235).
2512 - Added support for locale-specific integer formatting with the `n`
2513 specifier (https://github.com/fmtlib/fmt/issues/305):
2516 std::setlocale(LC_ALL, "en_US.utf8");
2517 fmt::print("cppformat: {:n}\n", 1234567); // prints 1,234,567
2520 - Sign is now preserved when formatting an integer with an incorrect
2521 `printf` format specifier
2522 (https://github.com/fmtlib/fmt/issues/265):
2525 fmt::printf("%lld", -42); // prints -42
2528 Note that it would be an undefined behavior in `std::printf`.
2530 - Length modifiers such as `ll` are now optional in printf formatting
2531 functions and the correct type is determined automatically
2532 (https://github.com/fmtlib/fmt/issues/255):
2535 fmt::printf("%d", std::numeric_limits<long long>::max());
2538 Note that it would be an undefined behavior in `std::printf`.
2540 - Added initial support for custom formatters
2541 (https://github.com/fmtlib/fmt/issues/231).
2543 - Fixed detection of user-defined literal support on Intel C++
2544 compiler (https://github.com/fmtlib/fmt/issues/311,
2545 https://github.com/fmtlib/fmt/pull/312).
2546 Thanks @dean0x7d and @speth.
2548 - Reduced compile time
2549 (https://github.com/fmtlib/fmt/pull/243,
2550 https://github.com/fmtlib/fmt/pull/249,
2551 https://github.com/fmtlib/fmt/issues/317):
2553 
2555 
2559 - Compile test fixes (https://github.com/fmtlib/fmt/pull/313).
2562 - Documentation fixes (https://github.com/fmtlib/fmt/pull/239,
2563 https://github.com/fmtlib/fmt/issues/248,
2564 https://github.com/fmtlib/fmt/issues/252,
2565 https://github.com/fmtlib/fmt/pull/258,
2566 https://github.com/fmtlib/fmt/issues/260,
2567 https://github.com/fmtlib/fmt/issues/301,
2568 https://github.com/fmtlib/fmt/pull/309).
2569 Thanks @ReadmeCritic @Gachapen and @jwilk.
2571 - Fixed compiler and sanitizer warnings
2572 (https://github.com/fmtlib/fmt/issues/244,
2573 https://github.com/fmtlib/fmt/pull/256,
2574 https://github.com/fmtlib/fmt/pull/259,
2575 https://github.com/fmtlib/fmt/issues/263,
2576 https://github.com/fmtlib/fmt/issues/274,
2577 https://github.com/fmtlib/fmt/pull/277,
2578 https://github.com/fmtlib/fmt/pull/286,
2579 https://github.com/fmtlib/fmt/issues/291,
2580 https://github.com/fmtlib/fmt/issues/296,
2581 https://github.com/fmtlib/fmt/issues/308).
2582 Thanks @mwinterb, @pweiskircher and @Naios.
2584 - Improved compatibility with Windows Store apps
2585 (https://github.com/fmtlib/fmt/issues/280,
2586 https://github.com/fmtlib/fmt/pull/285) Thanks @mwinterb.
2588 - Added tests of compatibility with older C++ standards
2589 (https://github.com/fmtlib/fmt/pull/273). Thanks @niosHD.
2591 - Fixed Android build
2592 (https://github.com/fmtlib/fmt/pull/271). Thanks @newnon.
2594 - Changed `ArgMap` to be backed by a vector instead of a map.
2595 (https://github.com/fmtlib/fmt/issues/261,
2596 https://github.com/fmtlib/fmt/pull/262). Thanks @mwinterb.
2598 - Added `fprintf` overload that writes to a `std::ostream`
2599 (https://github.com/fmtlib/fmt/pull/251).
2600 Thanks @nickhutchinson.
2602 - Export symbols when building a Windows DLL
2603 (https://github.com/fmtlib/fmt/pull/245).
2606 - Fixed compilation on Cygwin
2607 (https://github.com/fmtlib/fmt/issues/304).
2609 - Implemented a workaround for a bug in Apple LLVM version 4.2 of
2610 clang (https://github.com/fmtlib/fmt/issues/276).
2612 - Implemented a workaround for Google Test bug
2613 https://github.com/google/googletest/issues/705 on gcc 6
2614 (https://github.com/fmtlib/fmt/issues/268). Thanks @octoploid.
2616 - Removed Biicode support because the latter has been discontinued.
2618 # 2.1.1 - 2016-04-11
2620 - The install location for generated CMake files is now configurable
2621 via the `FMT_CMAKE_DIR` CMake variable
2622 (https://github.com/fmtlib/fmt/pull/299). Thanks @niosHD.
2623 - Documentation fixes
2624 (https://github.com/fmtlib/fmt/issues/252).
2626 # 2.1.0 - 2016-03-21
2628 - Project layout and build system improvements
2629 (https://github.com/fmtlib/fmt/pull/267):
2631 - The code have been moved to the `cppformat` directory. Including
2632 `format.h` from the top-level directory is deprecated but works
2633 via a proxy header which will be removed in the next major
2635 - C++ Format CMake targets now have proper interface definitions.
2636 - Installed version of the library now supports the header-only
2638 - Targets `doc`, `install`, and `test` are now disabled if C++
2639 Format is included as a CMake subproject. They can be enabled by
2640 setting `FMT_DOC`, `FMT_INSTALL`, and `FMT_TEST` in the parent
2645 # 2.0.1 - 2016-03-13
2647 - Improved CMake find and package support
2648 (https://github.com/fmtlib/fmt/issues/264). Thanks @niosHD.
2649 - Fix compile error with Android NDK and mingw32
2650 (https://github.com/fmtlib/fmt/issues/241). Thanks @Gachapen.
2651 - Documentation fixes
2652 (https://github.com/fmtlib/fmt/issues/248,
2653 https://github.com/fmtlib/fmt/issues/260).
2655 # 2.0.0 - 2015-12-01
2659 - \[Breaking\] Named arguments
2660 (https://github.com/fmtlib/fmt/pull/169,
2661 https://github.com/fmtlib/fmt/pull/173,
2662 https://github.com/fmtlib/fmt/pull/174):
2665 fmt::print("The answer is {answer}.", fmt::arg("answer", 42));
2670 - \[Experimental\] User-defined literals for format and named
2671 arguments (https://github.com/fmtlib/fmt/pull/204,
2672 https://github.com/fmtlib/fmt/pull/206,
2673 https://github.com/fmtlib/fmt/pull/207):
2676 using namespace fmt::literals;
2677 fmt::print("The answer is {answer}.", "answer"_a=42);
2682 - \[Breaking\] Formatting of more than 16 arguments is now supported
2683 when using variadic templates
2684 (https://github.com/fmtlib/fmt/issues/141). Thanks @Shauren.
2686 - Runtime width specification
2687 (https://github.com/fmtlib/fmt/pull/168):
2690 fmt::format("{0:{1}}", 42, 5); // gives " 42"
2695 - \[Breaking\] Enums are now formatted with an overloaded
2696 `std::ostream` insertion operator (`operator<<`) if available
2697 (https://github.com/fmtlib/fmt/issues/232).
2699 - \[Breaking\] Changed default `bool` format to textual, \"true\" or
2700 \"false\" (https://github.com/fmtlib/fmt/issues/170):
2703 fmt::print("{}", true); // prints "true"
2706 To print `bool` as a number use numeric format specifier such as
2710 fmt::print("{:d}", true); // prints "1"
2713 - `fmt::printf` and `fmt::sprintf` now support formatting of `bool`
2714 with the `%s` specifier giving textual output, \"true\" or \"false\"
2715 (https://github.com/fmtlib/fmt/pull/223):
2718 fmt::printf("%s", true); // prints "true"
2723 - \[Breaking\] `signed char` and `unsigned char` are now formatted as
2725 (https://github.com/fmtlib/fmt/pull/217).
2727 - \[Breaking\] Pointers to C strings can now be formatted with the `p`
2728 specifier (https://github.com/fmtlib/fmt/pull/223):
2731 fmt::print("{:p}", "test"); // prints pointer value
2736 - \[Breaking\] `fmt::printf` and `fmt::sprintf` now print null
2737 pointers as `(nil)` and null strings as `(null)` for consistency
2738 with glibc (https://github.com/fmtlib/fmt/pull/226).
2741 - \[Breaking\] `fmt::(s)printf` now supports formatting of objects of
2742 user-defined types that provide an overloaded `std::ostream`
2743 insertion operator (`operator<<`)
2744 (https://github.com/fmtlib/fmt/issues/201):
2747 fmt::printf("The date is %s", Date(2012, 12, 9));
2750 - \[Breaking\] The `Buffer` template is now part of the public API and
2751 can be used to implement custom memory buffers
2752 (https://github.com/fmtlib/fmt/issues/140). Thanks @polyvertex.
2754 - \[Breaking\] Improved compatibility between `BasicStringRef` and
2755 [std::experimental::basic_string_view](
2756 http://en.cppreference.com/w/cpp/experimental/basic_string_view)
2757 (https://github.com/fmtlib/fmt/issues/100,
2758 https://github.com/fmtlib/fmt/issues/159,
2759 https://github.com/fmtlib/fmt/issues/183):
2761 - Comparison operators now compare string content, not pointers
2762 - `BasicStringRef::c_str` replaced by `BasicStringRef::data`
2763 - `BasicStringRef` is no longer assumed to be null-terminated
2765 References to null-terminated strings are now represented by a new
2766 class, `BasicCStringRef`.
2768 - Dependency on pthreads introduced by Google Test is now optional
2769 (https://github.com/fmtlib/fmt/issues/185).
2771 - New CMake options `FMT_DOC`, `FMT_INSTALL` and `FMT_TEST` to control
2772 generation of `doc`, `install` and `test` targets respectively, on
2773 by default (https://github.com/fmtlib/fmt/issues/197,
2774 https://github.com/fmtlib/fmt/issues/198,
2775 https://github.com/fmtlib/fmt/issues/200). Thanks @maddinat0r.
2777 - `noexcept` is now used when compiling with MSVC2015
2778 (https://github.com/fmtlib/fmt/pull/215). Thanks @dmkrepo.
2780 - Added an option to disable use of `windows.h` when
2781 `FMT_USE_WINDOWS_H` is defined as 0 before including `format.h`
2782 (https://github.com/fmtlib/fmt/issues/171). Thanks @alfps.
2784 - \[Breaking\] `windows.h` is now included with `NOMINMAX` unless
2785 `FMT_WIN_MINMAX` is defined. This is done to prevent breaking code
2786 using `std::min` and `std::max` and only affects the header-only
2787 configuration (https://github.com/fmtlib/fmt/issues/152,
2788 https://github.com/fmtlib/fmt/pull/153,
2789 https://github.com/fmtlib/fmt/pull/154). Thanks @DevO2012.
2791 - Improved support for custom character types
2792 (https://github.com/fmtlib/fmt/issues/171). Thanks @alfps.
2794 - Added an option to disable use of IOStreams when `FMT_USE_IOSTREAMS`
2795 is defined as 0 before including `format.h`
2796 (https://github.com/fmtlib/fmt/issues/205,
2797 https://github.com/fmtlib/fmt/pull/208). Thanks @JodiTheTigger.
2799 - Improved detection of `isnan`, `isinf` and `signbit`.
2803 - Made formatting of user-defined types more efficient with a custom
2804 stream buffer (https://github.com/fmtlib/fmt/issues/92,
2805 https://github.com/fmtlib/fmt/pull/230). Thanks @NotImplemented.
2806 - Further improved performance of `fmt::Writer` on integer formatting
2807 and fixed a minor regression. Now it is \~7% faster than
2808 `karma::generate` on Karma\'s benchmark
2809 (https://github.com/fmtlib/fmt/issues/186).
2810 - \[Breaking\] Reduced [compiled code
2811 size](https://github.com/fmtlib/fmt#compile-time-and-code-bloat)
2812 (https://github.com/fmtlib/fmt/issues/143,
2813 https://github.com/fmtlib/fmt/pull/149).
2817 - \[Breaking\] Headers are now installed in
2818 `${CMAKE_INSTALL_PREFIX}/include/cppformat`
2819 (https://github.com/fmtlib/fmt/issues/178). Thanks @jackyf.
2821 - \[Breaking\] Changed the library name from `format` to `cppformat`
2822 for consistency with the project name and to avoid potential
2823 conflicts (https://github.com/fmtlib/fmt/issues/178).
2826 - C++ Format is now available in [Debian](https://www.debian.org/)
2828 ([stretch](https://packages.debian.org/source/stretch/cppformat),
2829 [sid](https://packages.debian.org/source/sid/cppformat)) and derived
2830 distributions such as
2831 [Ubuntu](https://launchpad.net/ubuntu/+source/cppformat) 15.10 and
2832 later (https://github.com/fmtlib/fmt/issues/155):
2834 $ sudo apt-get install libcppformat1-dev
2838 - [Packages for Fedora and
2839 RHEL](https://admin.fedoraproject.org/pkgdb/package/cppformat/) are
2840 now available. Thanks Dave Johansen.
2842 - C++ Format can now be installed via [Homebrew](http://brew.sh/) on
2843 OS X (https://github.com/fmtlib/fmt/issues/157):
2845 $ brew install cppformat
2847 Thanks @ortho and Anatoliy Bulukin.
2851 - Migrated from ReadTheDocs to GitHub Pages for better responsiveness
2852 and reliability (https://github.com/fmtlib/fmt/issues/128).
2853 New documentation address is <http://cppformat.github.io/>.
2854 - Added [Building thedocumentation](
2855 https://fmt.dev/2.0.0/usage.html#building-the-documentation)
2856 section to the documentation.
2857 - Documentation build script is now compatible with Python 3 and newer
2858 pip versions. (https://github.com/fmtlib/fmt/pull/189,
2859 https://github.com/fmtlib/fmt/issues/209).
2860 Thanks @JodiTheTigger and @xentec.
2861 - Documentation fixes and improvements
2862 (https://github.com/fmtlib/fmt/issues/36,
2863 https://github.com/fmtlib/fmt/issues/75,
2864 https://github.com/fmtlib/fmt/issues/125,
2865 https://github.com/fmtlib/fmt/pull/160,
2866 https://github.com/fmtlib/fmt/pull/161,
2867 https://github.com/fmtlib/fmt/issues/162,
2868 https://github.com/fmtlib/fmt/issues/165,
2869 https://github.com/fmtlib/fmt/issues/210).
2871 - Fixed out-of-tree documentation build
2872 (https://github.com/fmtlib/fmt/issues/177). Thanks @jackyf.
2876 - Fixed `initializer_list` detection
2877 (https://github.com/fmtlib/fmt/issues/136). Thanks @Gachapen.
2879 - \[Breaking\] Fixed formatting of enums with numeric format
2880 specifiers in `fmt::(s)printf`
2881 (https://github.com/fmtlib/fmt/issues/131,
2882 https://github.com/fmtlib/fmt/issues/139):
2885 enum { ANSWER = 42 };
2886 fmt::printf("%d", ANSWER);
2891 - Improved compatibility with old versions of MinGW
2892 (https://github.com/fmtlib/fmt/issues/129,
2893 https://github.com/fmtlib/fmt/pull/130,
2894 https://github.com/fmtlib/fmt/issues/132). Thanks @cstamford.
2896 - Fixed a compile error on MSVC with disabled exceptions
2897 (https://github.com/fmtlib/fmt/issues/144).
2899 - Added a workaround for broken implementation of variadic templates
2900 in MSVC2012 (https://github.com/fmtlib/fmt/issues/148).
2902 - Placed the anonymous namespace within `fmt` namespace for the
2903 header-only configuration (https://github.com/fmtlib/fmt/issues/171).
2906 - Fixed issues reported by Coverity Scan
2907 (https://github.com/fmtlib/fmt/issues/187,
2908 https://github.com/fmtlib/fmt/issues/192).
2910 - Implemented a workaround for a name lookup bug in MSVC2010
2911 (https://github.com/fmtlib/fmt/issues/188).
2913 - Fixed compiler warnings
2914 (https://github.com/fmtlib/fmt/issues/95,
2915 https://github.com/fmtlib/fmt/issues/96,
2916 https://github.com/fmtlib/fmt/pull/114,
2917 https://github.com/fmtlib/fmt/issues/135,
2918 https://github.com/fmtlib/fmt/issues/142,
2919 https://github.com/fmtlib/fmt/issues/145,
2920 https://github.com/fmtlib/fmt/issues/146,
2921 https://github.com/fmtlib/fmt/issues/158,
2922 https://github.com/fmtlib/fmt/issues/163,
2923 https://github.com/fmtlib/fmt/issues/175,
2924 https://github.com/fmtlib/fmt/issues/190,
2925 https://github.com/fmtlib/fmt/pull/191,
2926 https://github.com/fmtlib/fmt/issues/194,
2927 https://github.com/fmtlib/fmt/pull/196,
2928 https://github.com/fmtlib/fmt/issues/216,
2929 https://github.com/fmtlib/fmt/pull/218,
2930 https://github.com/fmtlib/fmt/pull/220,
2931 https://github.com/fmtlib/fmt/pull/229,
2932 https://github.com/fmtlib/fmt/issues/233,
2933 https://github.com/fmtlib/fmt/issues/234,
2934 https://github.com/fmtlib/fmt/pull/236,
2935 https://github.com/fmtlib/fmt/issues/281,
2936 https://github.com/fmtlib/fmt/issues/289).
2937 Thanks @seanmiddleditch, @dixlorenz, @CarterLi, @Naios, @fmatthew5876,
2938 @LevskiWeng, @rpopescu, @gabime, @cubicool, @jkflying, @LogicalKnight,
2939 @inguin and @Jopie64.
2941 - Fixed portability issues (mostly causing test failures) on ARM,
2942 ppc64, ppc64le, s390x and SunOS 5.11 i386
2943 (https://github.com/fmtlib/fmt/issues/138,
2944 https://github.com/fmtlib/fmt/issues/179,
2945 https://github.com/fmtlib/fmt/issues/180,
2946 https://github.com/fmtlib/fmt/issues/202,
2947 https://github.com/fmtlib/fmt/issues/225, [Red Hat Bugzilla
2948 Bug 1260297](https://bugzilla.redhat.com/show_bug.cgi?id=1260297)).
2949 Thanks @Naios, @jackyf and Dave Johansen.
2951 - Fixed a name conflict with macro `free` defined in `crtdbg.h` when
2952 `_CRTDBG_MAP_ALLOC` is set (https://github.com/fmtlib/fmt/issues/211).
2954 - Fixed shared library build on OS X
2955 (https://github.com/fmtlib/fmt/pull/212). Thanks @dean0x7d.
2957 - Fixed an overload conflict on MSVC when `/Zc:wchar_t-` option is
2958 specified (https://github.com/fmtlib/fmt/pull/214).
2961 - Improved compatibility with MSVC 2008
2962 (https://github.com/fmtlib/fmt/pull/236). Thanks @Jopie64.
2964 - Improved compatibility with bcc32
2965 (https://github.com/fmtlib/fmt/issues/227).
2967 - Fixed `static_assert` detection on Clang
2968 (https://github.com/fmtlib/fmt/pull/228). Thanks @dean0x7d.
2970 # 1.1.0 - 2015-03-06
2972 - Added `BasicArrayWriter`, a class template that provides operations
2973 for formatting and writing data into a fixed-size array
2974 (https://github.com/fmtlib/fmt/issues/105 and
2975 https://github.com/fmtlib/fmt/issues/122):
2979 fmt::ArrayWriter w(buffer);
2980 w.write("The answer is {}", 42);
2983 - Added [0 A.D.](http://play0ad.com/) and [PenUltima Online
2984 (POL)](http://www.polserver.com/) to the list of notable projects
2987 - C++ Format now uses MSVC intrinsics for better formatting performance
2988 (https://github.com/fmtlib/fmt/pull/115,
2989 https://github.com/fmtlib/fmt/pull/116,
2990 https://github.com/fmtlib/fmt/pull/118 and
2991 https://github.com/fmtlib/fmt/pull/121). Previously these
2992 optimizations where only used on GCC and Clang.
2993 Thanks @CarterLi and @objectx.
2995 - CMake install target
2996 (https://github.com/fmtlib/fmt/pull/119). Thanks @TrentHouliston.
2998 You can now install C++ Format with `make install` command.
3000 - Improved [Biicode](http://www.biicode.com/) support
3001 (https://github.com/fmtlib/fmt/pull/98 and
3002 https://github.com/fmtlib/fmt/pull/104).
3003 Thanks @MariadeAnton and @franramirez688.
3005 - Improved support for building with [Android NDK](
3006 https://developer.android.com/tools/sdk/ndk/index.html)
3007 (https://github.com/fmtlib/fmt/pull/107). Thanks @newnon.
3009 The [android-ndk-example](https://github.com/fmtlib/android-ndk-example)
3010 repository provides and example of using C++ Format with Android NDK:
3012 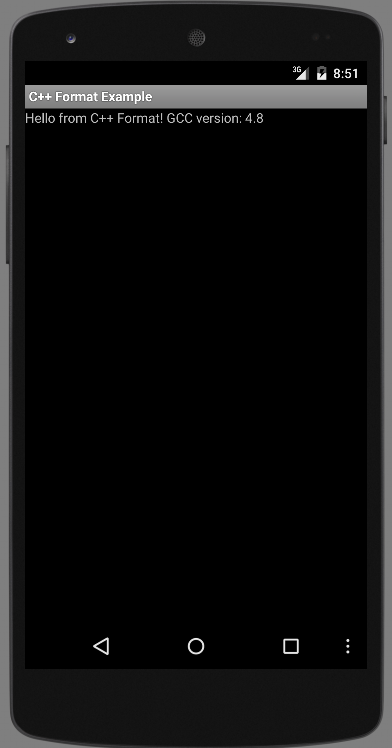
3014 - Improved documentation of `SystemError` and `WindowsError`
3015 (https://github.com/fmtlib/fmt/issues/54).
3017 - Various code improvements
3018 (https://github.com/fmtlib/fmt/pull/110,
3019 https://github.com/fmtlib/fmt/pull/111
3020 https://github.com/fmtlib/fmt/pull/112). Thanks @CarterLi.
3022 - Improved compile-time errors when formatting wide into narrow
3023 strings (https://github.com/fmtlib/fmt/issues/117).
3025 - Fixed `BasicWriter::write` without formatting arguments when C++11
3027 (https://github.com/fmtlib/fmt/issues/109).
3029 - Fixed header-only build on OS X with GCC 4.9
3030 (https://github.com/fmtlib/fmt/issues/124).
3032 - Fixed packaging issues (https://github.com/fmtlib/fmt/issues/94).
3034 - Added [changelog](https://github.com/fmtlib/fmt/blob/master/ChangeLog.md)
3035 (https://github.com/fmtlib/fmt/issues/103).
3037 # 1.0.0 - 2015-02-05
3039 - Add support for a header-only configuration when `FMT_HEADER_ONLY`
3040 is defined before including `format.h`:
3043 #define FMT_HEADER_ONLY
3047 - Compute string length in the constructor of `BasicStringRef` instead
3048 of the `size` method
3049 (https://github.com/fmtlib/fmt/issues/79). This eliminates
3050 size computation for string literals on reasonable optimizing
3053 - Fix formatting of types with overloaded `operator <<` for
3054 `std::wostream` (https://github.com/fmtlib/fmt/issues/86):
3057 fmt::format(L"The date is {0}", Date(2012, 12, 9));
3060 - Fix linkage of tests on Arch Linux
3061 (https://github.com/fmtlib/fmt/issues/89).
3063 - Allow precision specifier for non-float arguments
3064 (https://github.com/fmtlib/fmt/issues/90):
3067 fmt::print("{:.3}\n", "Carpet"); // prints "Car"
3070 - Fix build on Android NDK (https://github.com/fmtlib/fmt/issues/93).
3072 - Improvements to documentation build procedure.
3074 - Remove `FMT_SHARED` CMake variable in favor of standard [BUILD_SHARED_LIBS](
3075 http://www.cmake.org/cmake/help/v3.0/variable/BUILD_SHARED_LIBS.html).
3077 - Fix error handling in `fmt::fprintf`.
3079 - Fix a number of warnings.
3081 # 0.12.0 - 2014-10-25
3083 - \[Breaking\] Improved separation between formatting and buffer
3084 management. `Writer` is now a base class that cannot be instantiated
3085 directly. The new `MemoryWriter` class implements the default buffer
3086 management with small allocations done on stack. So `fmt::Writer`
3087 should be replaced with `fmt::MemoryWriter` in variable
3099 fmt::MemoryWriter w;
3102 If you pass `fmt::Writer` by reference, you can continue to do so:
3105 void f(fmt::Writer &w);
3108 This doesn\'t affect the formatting API.
3110 - Support for custom memory allocators
3111 (https://github.com/fmtlib/fmt/issues/69)
3113 - Formatting functions now accept [signed char]{.title-ref} and
3114 [unsigned char]{.title-ref} strings as arguments
3115 (https://github.com/fmtlib/fmt/issues/73):
3118 auto s = format("GLSL version: {}", glGetString(GL_VERSION));
3121 - Reduced code bloat. According to the new [benchmark
3122 results](https://github.com/fmtlib/fmt#compile-time-and-code-bloat),
3123 cppformat is close to `printf` and by the order of magnitude better
3124 than Boost Format in terms of compiled code size.
3126 - Improved appearance of the documentation on mobile by using the
3128 theme](http://ryan-roemer.github.io/sphinx-bootstrap-theme/):
3132 |  |  |
3134 # 0.11.0 - 2014-08-21
3136 - Safe printf implementation with a POSIX extension for positional
3140 fmt::printf("Elapsed time: %.2f seconds", 1.23);
3141 fmt::printf("%1$s, %3$d %2$s", weekday, month, day);
3144 - Arguments of `char` type can now be formatted as integers (Issue
3145 https://github.com/fmtlib/fmt/issues/55):
3148 fmt::format("0x{0:02X}", 'a');
3151 - Deprecated parts of the API removed.
3153 - The library is now built and tested on MinGW with Appveyor in
3154 addition to existing test platforms Linux/GCC, OS X/Clang,
3157 # 0.10.0 - 2014-07-01
3161 - All formatting methods are now implemented as variadic functions
3162 instead of using `operator<<` for feeding arbitrary arguments into a
3163 temporary formatter object. This works both with C++11 where
3164 variadic templates are used and with older standards where variadic
3165 functions are emulated by providing lightweight wrapper functions
3166 defined with the `FMT_VARIADIC` macro. You can use this macro for
3167 defining your own portable variadic functions:
3170 void report_error(const char *format, const fmt::ArgList &args) {
3171 fmt::print("Error: {}");
3172 fmt::print(format, args);
3174 FMT_VARIADIC(void, report_error, const char *)
3176 report_error("file not found: {}", path);
3179 Apart from a more natural syntax, this also improves performance as
3180 there is no need to construct temporary formatter objects and
3181 control arguments\' lifetimes. Because the wrapper functions are
3182 very lightweight, this doesn\'t cause code bloat even in pre-C++11
3185 - Simplified common case of formatting an `std::string`. Now it
3186 requires a single function call:
3189 std::string s = format("The answer is {}.", 42);
3192 Previously it required 2 function calls:
3195 std::string s = str(Format("The answer is {}.") << 42);
3198 Instead of unsafe `c_str` function, `fmt::Writer` should be used
3199 directly to bypass creation of `std::string`:
3203 w.write("The answer is {}.", 42);
3204 w.c_str(); // returns a C string
3207 This doesn\'t do dynamic memory allocation for small strings and is
3208 less error prone as the lifetime of the string is the same as for
3209 `std::string::c_str` which is well understood (hopefully).
3211 - Improved consistency in naming functions that are a part of the
3212 public API. Now all public functions are lowercase following the
3213 standard library conventions. Previously it was a combination of
3214 lowercase and CapitalizedWords. Issue
3215 https://github.com/fmtlib/fmt/issues/50.
3217 - Old functions are marked as deprecated and will be removed in the
3222 - Experimental support for printf format specifications (work in
3226 fmt::printf("The answer is %d.", 42);
3227 std::string s = fmt::sprintf("Look, a %s!", "string");
3230 - Support for hexadecimal floating point format specifiers `a` and
3234 print("{:a}", -42.0); // Prints -0x1.5p+5
3235 print("{:A}", -42.0); // Prints -0X1.5P+5
3238 - CMake option `FMT_SHARED` that specifies whether to build format as
3239 a shared library (off by default).
3241 # 0.9.0 - 2014-05-13
3243 - More efficient implementation of variadic formatting functions.
3245 - `Writer::Format` now has a variadic overload:
3249 out.Format("Look, I'm {}!", "variadic");
3252 - For efficiency and consistency with other overloads, variadic
3253 overload of the `Format` function now returns `Writer` instead of
3254 `std::string`. Use the `str` function to convert it to
3258 std::string s = str(Format("Look, I'm {}!", "variadic"));
3261 - Replaced formatter actions with output sinks: `NoAction` -\>
3262 `NullSink`, `Write` -\> `FileSink`, `ColorWriter` -\>
3263 `ANSITerminalSink`. This improves naming consistency and shouldn\'t
3264 affect client code unless these classes are used directly which
3265 should be rarely needed.
3267 - Added `ThrowSystemError` function that formats a message and throws
3268 `SystemError` containing the formatted message and system-specific
3269 error description. For example, the following code
3272 FILE *f = fopen(filename, "r");
3274 ThrowSystemError(errno, "Failed to open file '{}'") << filename;
3277 will throw `SystemError` exception with description \"Failed to open
3278 file \'\<filename\>\': No such file or directory\" if file doesn\'t
3281 - Support for AppVeyor continuous integration platform.
3283 - `Format` now throws `SystemError` in case of I/O errors.
3285 - Improve test infrastructure. Print functions are now tested by
3286 redirecting the output to a pipe.
3288 # 0.8.0 - 2014-04-14